Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial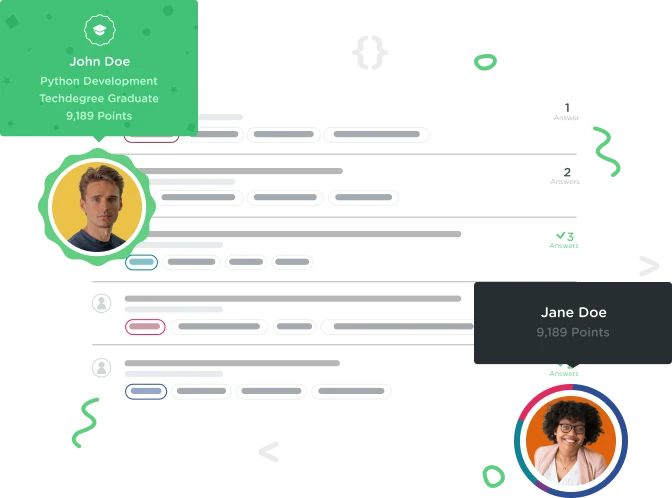
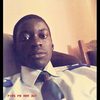
MUZ140934 Noel Zipingani
2,656 Pointshelp me complete this code challenge
Write a function named time_machine that takes an integer and a string of "minutes", "hours", "days", or "years". This describes a timedelta. Return a datetime that is the timedelta's duration from the starter datetime
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(integer,string):
if string == 'hours' :
return starter + datetime.timedelta(hours = integer)
elif string == 'days' :
return starter + datetime.timedelta(days = integer)
elif string == 'year' :
return starter + datetime.timedelta(year = integer*365)
elif string == 'minutes' :
return starter + datetime.timedelta(minutes = integer)
time_machine(3,'days')
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
5 Answers

Jing Zhang
Courses Plus Student 6,465 PointsI think this is a good place to use eval.
def time_machine(integer, string):
if string == 'years':
integer *= 365
string = "days"
return starter + eval("datetime.timedelta({}=integer)".format(string))
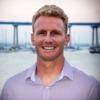
BRIAN WEBER
21,570 PointsMy apologies. I was trying to match up the days when I changed the years. I got the code to work by removing the + 1 and by changing 'year' to 'years'.
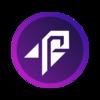
Rocket Dollar Invest
7,129 PointsA simple solution for this problem is to first check if the string is 'years' and if so change the string to 'days' since timedelta will not except years as an argument. Then multiply the integer by 365 to get the equivalent number of days for the given number of years. Finally, return the duration as the difference of the starter datetime and the timedelta of the string and integer passed to the method as a literal dictionary with the ** prefix operator to unpack the dictionary.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(integer, string):
if string == 'years':
string = 'days'
integer *= 365
return starter + datetime.timedelta(**{string: integer})
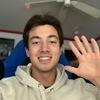
Kerrick Hahn
5,728 PointsDoes this work because Python automatically unpacks libraries as key=value? Thus passing key=value as the argument to datetime.timedelta() ?
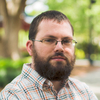
Kenneth Love
Treehouse Guest TeacherYou can't do timedelta(year=9)
. So you have to make a year. Years are made up of days, so how would you go from a provided number, like 9, and the attribute days
, to get 9 years? Remember, years should just be considered as 365 days.
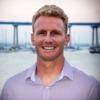
BRIAN WEBER
21,570 PointsKenneth this is the code that I got which outputs all of the correct timedeltas, even the years. But for some reason I am not Code Challenge is not accepting the answer. Can you give me a further hint on what I am doing wrong? Thanks!
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(integer,string):
if string == 'hours' :
return starter + datetime.timedelta(hours = integer)
elif string == 'days' :
return starter + datetime.timedelta(days = integer)
elif string == 'year' :
return starter + datetime.timedelta(days = integer * 365 + 1)
elif string == 'minutes' :
return starter + datetime.timedelta(minutes = integer)
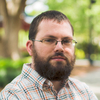
Kenneth Love
Treehouse Guest TeacherWhy are you adding 1 for the year?
Also, you need to check for "years"
, not "year"
.

Clayton Sibanda
5,044 PointsIsn't there other short method for solving this challenge
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherI don't think there's ever a good place to use
eval
. :)That said, your solution isn't horrible. I still wouldn't use
eval
there, though. You can use dict unpacking to make a much cleaner, safer version.