Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial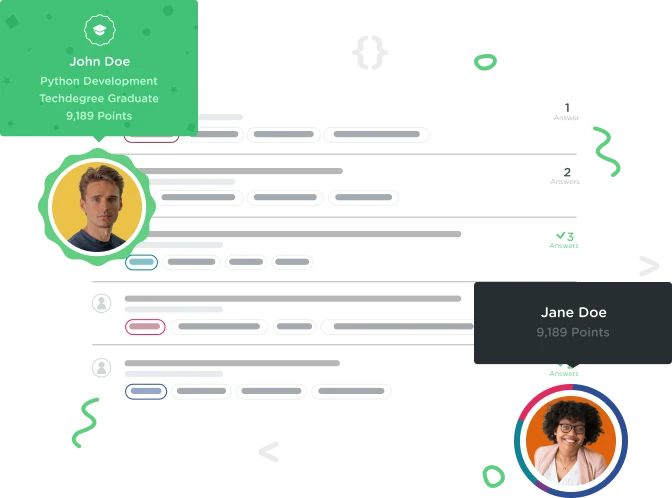

shawn khah
15,082 Pointshelp plz
Challenge Task 1 of 1
Add a static method named CalculateHypotenuse to the RightTriangle class that returns the length of long side of a right triangle (the hypotenuse), given the length of each of the the two shorter sides (the legs). The Pythagorean theorem will come in handy. The method should take two parameters of type double and return a double. Important: In each task of this code challenge, the code you write should be added to the code from the previous task. Restart Preview Get Help Check Work RightTriangle.cs
1 namespace Treehouse.CodeChallenges 2 { 3 class RightTriangle 4 { 5
6 } 7 } 8 ā
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
}
}
3 Answers

Chris Adamson
132,143 PointsFor this exercise they are looking for a static method which calculates the third side of a triangle, using the pythagoreom theorem c squared = a squared + b squared and its equivalent, c = square_root(a squared + b square), you can use the Math library Sqrt to calculate the hypotenuse:
using System;
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
public static double CalculateHypotenuse(double a, double b) {
// c^2 = a^2 + b^2, or c = sqrt(a^2 + b^2)
return Math.Sqrt(a*a + b*b);
}
}
}

Chris Adamson
132,143 PointsAs you figured out, you can use the System namespace directly to access the Math.Sqrt function:
namespace Treehouse.CodeChallenges {
class RightTriangle {
public static double CalculateHypotenuse(double a, double b) {
return System.Math.Sqrt(a*a + b*b);
}
}
}
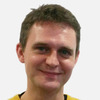
Joel Brennan
18,300 PointsThanks Chris.
I wonder if you may be able to advise me on this please?
Why do we not need to use System.Math.Sqrt() ?
If System is namespaced at the top is it not needed?
Regards
Jeremy McLain
Treehouse Guest TeacherJeremy McLain
Treehouse Guest TeacherWhat, specifically, would you like help with? Static methods? Pathagorean Theorem? Right triangles? Doubles?
What have you tried so far? You can post what you've tried and that may give us some idea of how to help you.