Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial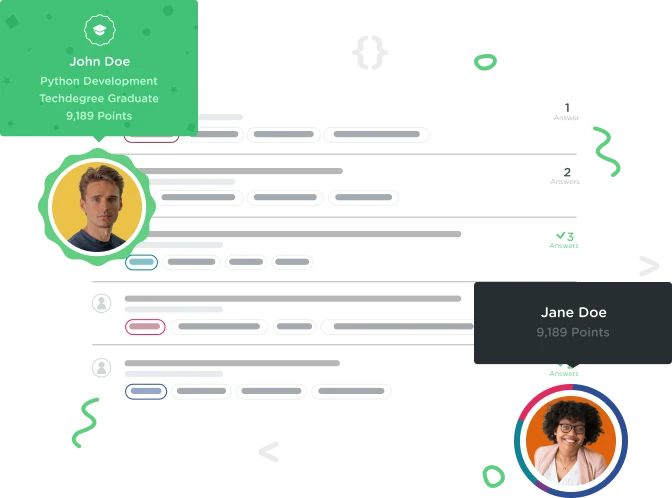

Mischa Potter
2,555 PointsHelp with my code(python)
I am learning python, and I decided to make a gunman file to practice my skills. I am stuck on one thing.
def add_gun_inv(item):
inventory.append(item)
print("You have added {} to your inventory.".format(pistol, shotgun))
When I run the code, it tells me:
TypeError: add_gun_inv() missing 1 required positional argument: 'item'
Please help! Btw the code is in the comments.
2 Answers

Daniel Polok
8,483 Pointsdef choose_gun():
gun_type = input("Which gun do you want? ")
if gun_type == "Pistol":
pistol = input("Nice, which type of pistol? ")
if pistol == "Glock":
add_gun_inv() #No 'item' argument
elif pistol == "Deagle":
add_gun_inv() #No 'item' argument
You want that the add_gun_function() take the item parameter but you forgot to pass the argument while calling the function. You should do that this way :
def choose_gun():
gun_type = input("Which gun do you want? ")
if gun_type == "Pistol":
pistol = input("Nice, which type of pistol? ")
if pistol == "Glock":
add_gun_inv("Glock")
elif pistol == "Deagle":
add_gun_inv("Deagle")
and then in the add_gun_inv() function:
def add_gun_inv(item):
inventory.append(item)
print("You have added {} to your inventory.".format(item))
If you have more question, I will help with pleasure.

Mischa Potter
2,555 Pointsinventory = []
bullets = 20
def show_actions():
print("Lets get guns and shoot them")
print("""
Enter 'GET GUN' to get a gun
Enter 'SHOW ACTIONS' to see this page
Enter 'SHOOT' to shoot
Enter 'INVENTORY' to see your inventory
Enter 'DONE' to stop
""")
def shoot():
confirm_shoot = input("Are you sure you want to shoot? ")
if confirm_shoot == "Yes":
if bullets >= 1:
bullets -= 1
print("You shot. You lost 1 bullet.")
elif bullets <= 1:
print("You didn't have enough bullets. You didn't shoot.")
def add_gun_inv(item):
inventory.append(item)
print("You have added {} to your inventory.".format(pistol, shotgun))
def show_inv():
print("This is what is in your inventory:")
for item in inventory:
print(item)
def choose_gun():
gun_type = input("Which gun do you want? ")
if gun_type == "Pistol":
pistol = input("Nice, which type of pistol? ")
if pistol == "Glock":
add_gun_inv()
elif pistol == "Deagle":
add_gun_inv()
elif gun_type == "Shotgun":
shotgun = input("Good choice. Which type? ")
if shotgun == "Double barel":
add_gun_inv()
show_actions()
while True:
game = input("> ")
if game == "DONE":
break
elif game == "GET GUN":
choose_gun()
continue
if game == "SHOOT":
shoot()
continue
elif game == "SHOW ACTIONS":
show_actions()
continue
if game == "INVENTORY":
show_inv()
continue
show_inv()

Mischa Potter
2,555 PointsThis is my code I didn't want to put it in the description because I thought it would be too big.
Mischa Potter
2,555 PointsMischa Potter
2,555 PointsThank you! I added this to my code and now it works ;) small disclaimer: I do not like shooting guns, and in fact have never shot one
Daniel Polok
8,483 PointsDaniel Polok
8,483 PointsNo problem : )