Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial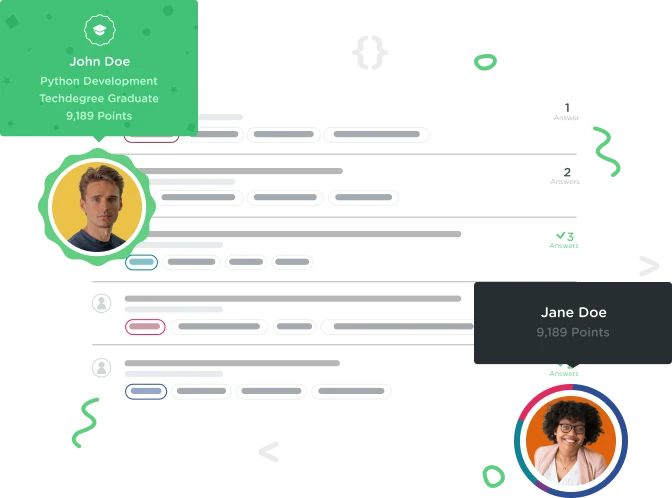
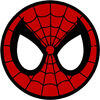
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsHey everyone I am sorry to bother you but I categorically do not understand how to get this to work.
I do not understand why this does not work. I am sorry to bother you with this but I think you guys need to redo these sets of videos and I don't think the language is simple enough. Anyway if someone could maybe help me understand how to sort this out that would be amazing.
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
get level(this.credits) {
if (this.credits >= 90) {
return 'Senior'
} else if (this.credits < 90 && this.credits >= 61) {
return 'Junior'
} else if (this.credits < 61 && this.credits >= 31) {
return 'Sophomore'
} else {
return 'Freshman'
}
}
}
stringGPA() {
return this.gpa.toString();
}
}
const student = new Student(3.9);
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
get level(this.credits) {
if (this.credits >= 90) {
return 'Senior'
} else if (this.credits < 90 && this.credits >= 61) {
return 'Junior'
} else if (this.credits < 61 && this.credits >= 31) {
return 'Sophomore'
} else {
return 'Freshman'
}
}
}
stringGPA() {
return this.gpa.toString();
}
}
const student = new Student(3.9);
Mod edit: added code formatting for readability. Check out the "markdown cheatsheet" linked below the comment box for tips on how to format your posts.
4 Answers
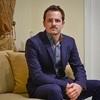
Nikos Papapetrou
6,305 PointsFirst you have some mess with the curly braces. You should set it after the method so you don't confuse. Also I am not sure if you have to pass argument in the get level. Make some try and see if it works.
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
} //end of constructor
stringGPA() {
return this.gpa.toString();
} //end of stringGPA
get level() {
if (this.credits >= 90) {
return 'Senior'
} else if (this.credits < 90 && this.credits >= 61) {
return 'Junior'
} else if (this.credits < 61 && this.credits >= 31) {
return 'Sophomore'
} else {
return 'Freshman'
}
} //end of get level
} //end of class
const student = new Student(3.9);
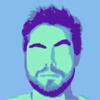
Cameron Childres
11,818 PointsHi Taylor,
Getter methods don't take arguments. Remove "this.credits" from the parentheses following get level
and the rest of your code will be able to run. There's also an extra curly brace after your getter method that you need to remove.
Once you've done that you'll run in to one other problem -- a student with 90 credits will be considered a senior. Reread the first two requirements in the challenge:
- If student has more than 90 credits, they are a 'Senior'.
- If the student has 90 or fewer credits, but more than 60 (>= 61), they are a 'Junior'.
Change your conditionals to match these requirements and you should be good to go! Let me know if you run in to any issues and I'll do my best to help out.
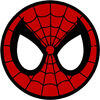
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsCameron Childres Nikos Papapetrou Thank you both so much for helping me I felt like I was loosing my mind.

Mat T'Qartit
29,277 PointsThis worked for me get level(){ if (this.credits > 90) { return 'Senior'
} else if (this.credits <= 90 && this.credits >= 61) {
return 'Junior'
} else if (this.credits <= 61 && this.credits >= 31) {
return 'Sophomore'
} else {
return 'Freshman'
}
}