Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial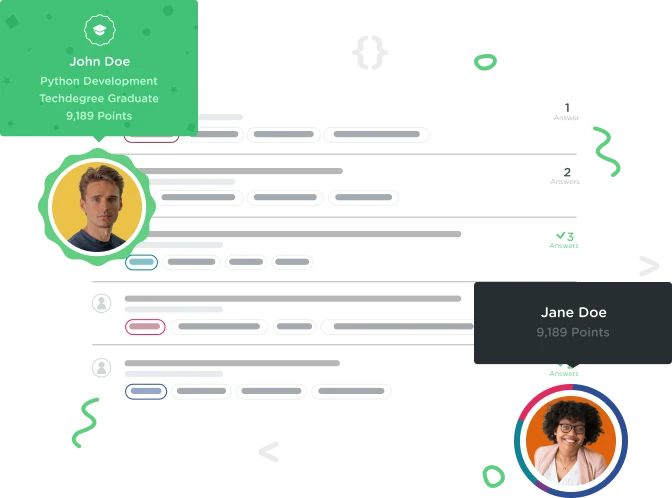

Federico Isabello
1,637 PointsHi!, I need to start the car in color red and afterwards I have to change it to blue, what is the problem in this code?
class Car():
def __init__(self, brand, color):
self.brand = brand
self.color = color
self.engine_started = False
def start_engine(self):
self.engine_started = True
def stop_engine(self):
self.engine_started = False
# Add a method below to change the color of a car
def ch_color(self, color):
self.color = color
Instantiate a red car and change it to blue
car_red = Car('brand', 'red')
car_red.__initiate__(self, 'brand', 'red')
car_blue = car_red.ch_color('brand', 'blue')
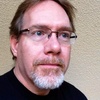
Chris Freeman
Treehouse Moderator 68,426 PointsPro tip: you can improve post readability by wrapping code in
```python and ```
using backticks (left of 1 on keyboard)
1 Answer

jb30
44,806 PointsWhen you run car_red = Car('brand', 'red')
, it runs the __init__
method in Car for you. When you run car_blue = car_red.ch_color('blue')
, car_red
changes its color to 'blue', then returns None, and car_blue
is set to None.
car_red = Car('brand', 'red')
car_red.ch_color('blue')

Federico Isabello
1,637 PointsThanks jb !
I change the code for the following, how can I change the return of None that you mention? In this code I tried to see if the blue_car is an instance of the class and return false, if it should be equal to red why is not part of the same class?
class Car():
def __init__(self, brand, color):
self.brand = brand
self.color = color
self.engine_started = False
def start_engine(self):
self.engine_started = True
def stop_engine(self):
self.engine_started = False
# Add a method below to change the color of a car
def ch_color(self, color):
self.color = color
Instantiate a red car and change it to blue
car_red = Car('brand', 'red') car_blue = car_red.ch_color('blue')
print(isinstance(car_blue, Car))

jb30
44,806 PointsIf you want ch_color to have a return value,
def ch_color(self, color):
self.color = color
return self
Federico Isabello
1,637 PointsFederico Isabello
1,637 PointsI think I got it!
Is the following code correct?
class Car():
Instantiate a red car and change it to blue
car_red = Car('brand', 'red')
car_red.init('brand', 'red')
car_blue = car_red.ch_color('blue')