Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial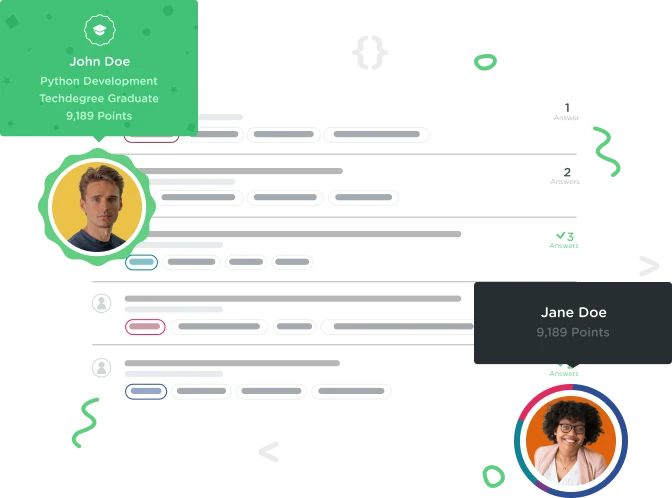

Shukran Ali
132 PointsHi in python if I want to create an input variable, why does it get excecuted without being called, thanks
I thought variables only store data so that it can be used in strings
4 Answers
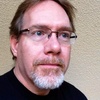
Chris Freeman
Treehouse Moderator 68,460 PointsWhile Python does have a type of lazy evaluation with respect to evaluation iterators, it does not apply to strings. When the code my_name = "Shukran"
is evaluated, immediately, the str
object "Shukran" is created in memory, and the label my_name
is pointed at this new object.
Variable names in Python are simply labels that point to objects in memory. Using the id()
function you can see that a label is just an address pointer.
In the case of the input
call evaluation, if it is not inside of a function or method, further execution is immediate halted until the input
function receives input from the user. If run in a context that does not allow the user to input a response, the program will hang indefinitely.
Also note, Python is a "pass object by reference" language, in that, reference labels ("variable names") are passed into functions instead of the object itself. When a function references a parameter, that parameter name (label) and the original object label both point to the actual object in memory. This is how a function may modify a mutable object outside of the function.
Post back if you have more questions. Good luck!!
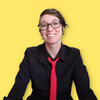
Mel Rumsey
Treehouse ModeratorHey Shukran Ali! Great question :) When input() function executes, the program stops until the user has given an input. So when you have a variable that has a value of input(), the programs stops until that input is entered and the variable has a value.
Ex.
name = input("What is your name? ")
When this comes up in the program, the program will stop completely until the user enters their name. Once that input has been received, the value of name is a string of whatever the user enters. In your case it would be name = "Shukran"
. The name variable now, no longer has an input as it's value and the program continues to run.
You can read all about Taking Input here! Hopefully, this helps!

Shukran Ali
132 PointsHi thanks, but my question was if i have a variable called my_name = "Shukran" it wont get executed until i use it in a print function, so why does the input variable get excecuted when i run the progamme without priting or calling it?
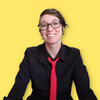
Mel Rumsey
Treehouse ModeratorHey Shukran Ali. Yes, I understand. The input function is being called if a variable is being created that has a value of input()
. Your program runs from the top down. If you have a variable at the top that equals an input, the program will stop until the value of that variable is set to whatever the input may be. Then the program will continue.

Shukran Ali
132 PointsYeah my question is why does it do that? Can you not just store it without it being excecuted like you would store a normal variable
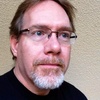
Chris Freeman
Treehouse Moderator 68,460 PointsNot sure what "store it without it being executed" means. If you mean delaying the call of the input
function. This would happen if the input
is inside of another function. Evaluating a function definition is not the same as executing a function call. Using input
outside of a function, is an executable statement. In this case, the input
would execute immediately.