Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial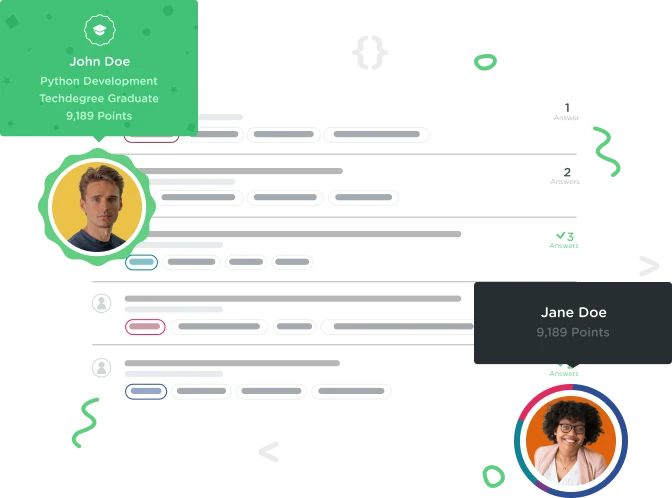

Quinten Rowland
1,310 Pointshow do I get my function to return "hello" followed by the name of someone as an argument, from my parameter of name
I don't know why this isn't working, it keeps saying that ashley isn't defined, but its supposed to be my argument for the parameter i set up so i don't understand.
def hello_student(name):
val = ("Hello")
return val
hello_student(ashley)
2 Answers
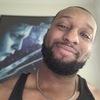
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi Quinten,
Two things. One, you have to pass a string into the function. This means you need to wrap ashley in quotation marks.
Two, in your function you can’t just return the val variable. You have to concatenate the val variable with the name parameter, and return that.
In your function you have name as your function’s parameter, but in the body of your function you never reference name.
Once you fix those two small issues, you’re golden.

Quinten Rowland
1,310 Pointsso i used your tips and got the correct answer, but i'm confused as to why i had to use Ashley for the val variable. I tried to use name, but it kept coming up saying that my string was incorrect. shouldn't i be able to do something like val = ("Hello", name) and then have any name satisfy the parameters?
def hello_student(name): val = ("Hello Ashley") return val hello_student("Ashley")
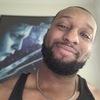
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 Pointsdef hello_student(name):
val = (“Hello Ashley”)
return val
hello_student(“Ashley”)
“””
given the way the function is written
it does not matter what name/string
you pass into it, because ‘Hello Ashley’
is hard coded to be the value returned
“””
The name
parameter is meant to be referenced within the code.
So say I want to write a function that squares a number, I might write it like this:
def square(number): # when the function is called an int will need to be passed in
return number * number # here is where I reference the number parameter
square(5) # output: 25
def square_hard_coded(number): # when the function is called an int will need to be passed in here as well
return 4 * 4 # the diff b/w this function and the one above is that no matter what, 16 will be returned
square_hard_coded(5) # output: 16 (even though 5 was passed in as an arg)
You don’t have to use ‘Ashley’ as the val variable. Ashley is the argument that Treehouse passed into your function as a test, and I think what happened is you read Bummer:AssertionError: (‘Hello’, ‘Ashley’) != ‘Hello Ashley’:
and took it as ‘Ashley’ needed to be the variable returned. But Ashley doesn’t need to be hard coded, and in fact shouldn’t be hard coded.
This statement: val = (“Hello”, name)
stores a tuple into the val variable, which means you’re returning a tuple with the statement: return val
instead of a string.
Concatenation can be done in a few different ways, but maybe the simplest to understand is new_string = string1 + string2
When working with numbers the the + operator adds multiple operands together.
When working with strings the + operator concatenates multiple strings together.
Does that make sense?