Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial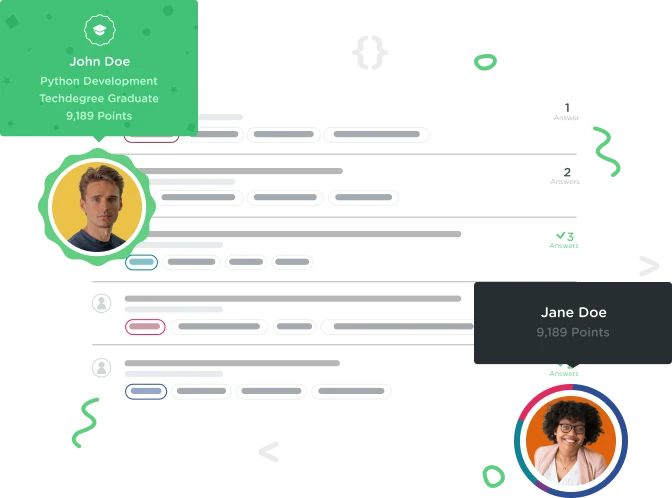

Michelle Wallerius
5,221 PointsHow do you make python objects appear as strings?
I'm working on the OOP challenge right now and I can't figure out what I'm doing wrong. The challenge is to create a memory game with a 4 x 4 grid. I am trying to assign each card a word and random location but when I checked my code with a print statement I get this"
[<cards.Card object at 0x7f973e468130>, <cards.Card object at 0x7f973e762d00>, <cards.Card o bject at 0x7f973e468160>, <cards.Card object at 0x7f973e762e20>, <cards.Card object at 0x7f9 73e762640>, <cards.Card object at 0x7f973e762760>, <cards.Card object at 0x7f973e7627c0>, <c ards.Card object at 0x7f973e766ac0>, <cards.Card object at 0x7f973e766a60>, <cards.Card obje ct at 0x7f973e78d250>, <cards.Card object at 0x7f973e766790>, <cards.Card object at 0x7f973e 78d850>, <cards.Card object at 0x7f973e78d7c0>, <cards.Card object at 0x7f973e78dc40>, <card s.Card object at 0x7f973e78dee0>, <cards.Card object at 0x7f973e78da60>]
This is my cards.py file:
class Card:
def __init__(self, word, location):
self.card = word
self.location = location
self.matched = False
def __eq__(self, other):
return self.card == other.card
def __str__(self):
return self.card
and this is what is in my game.py file
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev', 'Egg', 'Far', 'Gum', 'Hut']
self.columns = ["A", "B", "C", "D"]
self.cards = []
self.locations = []
for column in self.columns:
for i in range(1, self.size + 1):
self.locations.append(f'{column}{i}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
print(self.cards)
g1 = Game()
g1.set_cards()
All help is appreciated!
1 Answer

amandae
15,727 PointsI'm just getting into OOP, too, but the first thing I would try is to check on your string object override. I'm thinking it should look like this.
def __str__(self):
return str(self.card)
I hope that works! I'll be curious to know if I saw it correctly.