Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial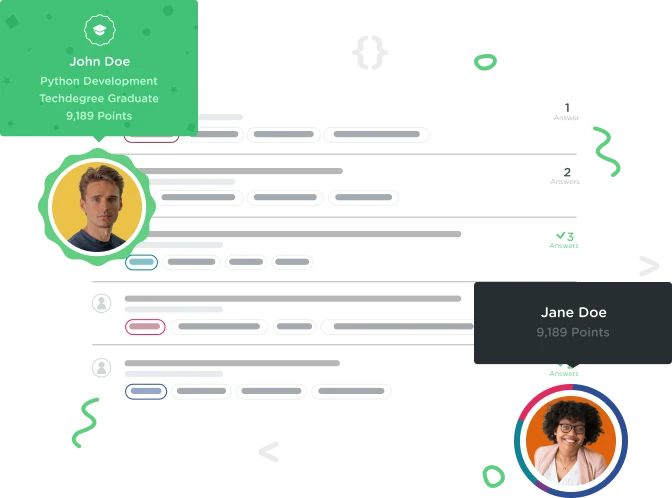
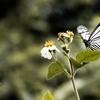
Justin Kao
5,724 PointsHow does Await function pause the entire Async function?
async function getPeopleInSpace (url) {
const peopleData = await getJson(url)
// assume peopleData is an array of 10 length
const profilesPromise = peopleData.people.map(async (person, i) => {
const craft = person.craft
console.log('profileintome'); // because of event loop, this line will log 10 times at a time
const profile = await getJson(wikiUrl + person.name)
console.log('profileinafterme', i,profile); // but why this line will show after await getJson
return {...profile, craft}
})
console.log('promiseprofile', profilesPromise);
const profiles = await Promise.all(profilesPromise)
generateHTML(profiles);
}
Below is console window
fetch successfully
2async-await.js:24 profileintome
8async-await.js:24 profileintome
async-await.js:29 promiseprofile Array(10)
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 0 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 6 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 2 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 7 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 8 Object
2async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 1 Object
async-await.js:26 profileinafterme 5 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 4 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 9 Object
async-await.js:10 fetch successfully
async-await.js:26 profileinafterme 3 Object
In the Async MDN, the example is the same.
async function asyncCall() {
console.log('calling');
const result = await resolveAfter2Seconds();
console.log(result); //
}
Here is my guess, I think every code after await, it will be put into a .then
Just want to make sure my concept is right or not.
2 Answers

JASON LEE
17,352 PointsOne thing that is helpful to understand from my experience is the blocking behavior of promises with respect to function scope. As an exercise, run this example in your code and try to predict the order of the console.log statements.
const promise1 = new Promise((resolve, reject) => {
setTimeout( ()=> {
resolve(`whatever resolve value here`)
}, 2000)
});
async function firstFunction() {
let result = await promise1; // <-- notice the await here
console.log(`Does this statement come first? `, result);
}
async function secondFunction() {
console.log(`Does this statement come last?`)
}
firstFunction();
secondFunction();