Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial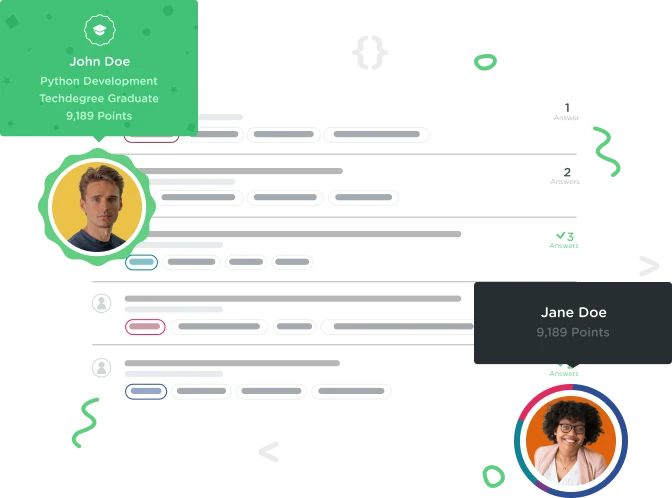

roshaan zafar
3,868 Pointshow to determine if user has pass the tile
cant make right algorithim
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
// TODO: Add the tile to tiles
this.tiles+="tiles";
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean hasTile=tiles.indexOf("tiles")==-1;
return hasTile;
}
}
2 Answers
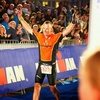
Steve Hunter
57,712 PointsHi Roshaan,
The first task is to add the parameter to the tiles
member variable. The parameter tile
is passed into the method. In there you want to use +=
to add tile
onto tiles
. You can use this
if you like, for greater readability. That looks like:
public void addTile(char tile) {
// TODO: Add the tile to tiles
tiles += tile;
}
The next part is seeing if tile
is in tiles
and you are so close!
You've correctly highlighted that you need to test using indexOf()
whether that's something to with -1
. Totally correct. The indexOf()
method will return -1
if tile
is not in tiles
. That expression would look like:
tiles.indexOf(tile)
That will evaluate to -1
if tile
isn't in tiles
. So, if we compare that to -1
, checking for equality, we get a true/false answer. Like this:
tiles.indexOf(tiles) == -1
That evaluates to true
if the result of the expression is -1
. That's not what we want. The instructions want that scenario to return false
. So, let's just negate the test. Rather than seeing if the expression == -1
let's see if it doesn't equal -1
- that's using !=
. The not-equal-to operator. That becomes tiles.indexOf(tile) != -1
So now we have an expression that evaluates to true
or false
, as required. We just need to return that from the method, right? How about:
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return tiles.indexOf(tile) != -1;
}
I hope that makes sense.
Steve.

roshaan zafar
3,868 Pointsthanks steve