Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial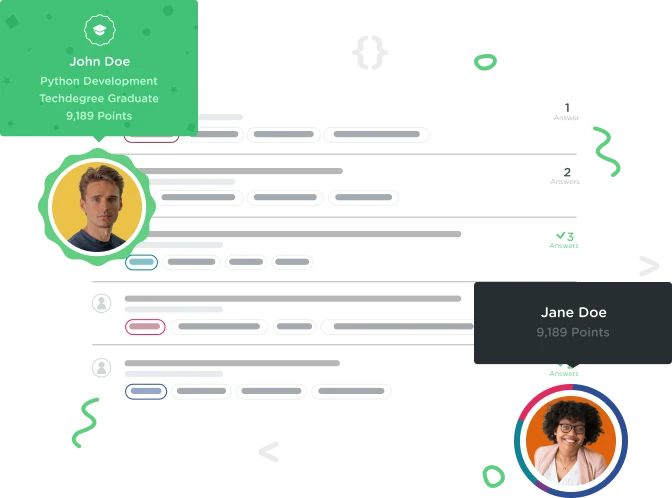

Ewen Kennedy
1,769 PointsHow to echo data from multidimensional arrays. How do I get the echo commands to display name and email
<?php //edit this array $contacts = array( array('name'=>'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'), array('name'=>'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'), array('name'=>'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'), array('name'=>'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com'), );
echo "<ul>\n"; //$contacts[0] will return 'Alena Holligan' in our simple array of names. echo "<li$contacts[0]</li>\n"; echo "<li>$contacts[1]</li>\n"; echo "<li>$contacts[2]</li>\n"; echo "<li>$contacts[3]</li>\n"; echo "</ul>\n";
<?php
//edit this array
$contacts = array(
array('name'=>'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'),
array('name'=>'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'),
array('name'=>'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'),
array('name'=>'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com'),
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li$contacts[0]</li>\n";
echo "<li>$contacts[1]</li>\n";
echo "<li>$contacts[2]</li>\n";
echo "<li>$contacts[3]</li>\n";
echo "</ul>\n";
1 Answer
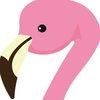
Dave StSomeWhere
19,870 PointsSince you have an array where each array element is another array (hence - multi dimensional), you'll need to provide index entries for each level of the array.
The $contacts Array in your example has 4 elements and they are numerically indexed starting at zero so Alena is index 0 and Dave is index 1 and so on...
At each index (0 thru 3) you have another array. An Associative array since they have named keys.
So, $contacts at index zero has a value for name and a value for email. You need to provide both indexes as per the example below:
<?php
// below is the second array in the $contacts array - Dave McFarland - since the index is 1
echo "<li>" . $contacts[1]['name'] . " the email is " . $contacts[1]['email'] ."<li>\n";
Test your code in your workspace or local environment.
Hope that helps