Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial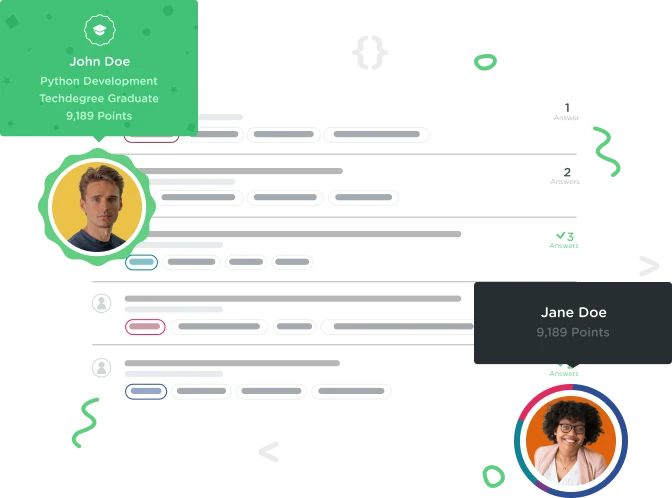

Jakub Mozdzierz
8,181 PointsHow to filter for dates in dataframe?
I have a pandas dataframe like this called data_daily and it is created using this code
ts = TimeSeries (key=api_key, output_format = "pandas")
data_daily, meta_data = ts.get_daily_adjusted(symbol=stock_ticker, outputsize ='full')
1. open 2. high ... 7. dividend amount 8. split coefficient
date ...
2020-09-21 325.7000 327.1300 ... 0.0000 1.0
2020-09-18 335.3700 335.4900 ... 1.3392 1.0
2020-09-17 333.5600 337.6996 ... 0.0000 1.0
2020-09-16 341.5100 343.0600 ... 0.0000 1.0
2020-09-15 341.1200 342.0200 ... 0.0000 1.0
... ... ... ... ... ...
1999-11-05 138.6250 139.1093 ... 0.0000 1.0
1999-11-04 136.7500 137.3593 ... 0.0000 1.0
1999-11-03 136.0000 136.3750 ... 0.0000 1.0
1999-11-02 135.9687 137.2500 ... 0.0000 1.0
1999-11-01 136.5000 137.0000 ... 0.0000 1.0
[5256 rows x 8 columns]
To iterate trough the rows and select date I had to use the word index. Not sure if this will help with finding the solution. I tried to ask on stack overflow but it has been a few days and nobody seems to know what is wrong haha
for index, row in data_daily.iterrows():
print(index, row['1. open'])
I want to set a date filters like this
start_date = '2000-01-01'
end_date = '2019-12-31'
number_of_years = end_date - start_date
This is my code for the main section
start_date = '2000-01-01'
end_date = '2019-12-31'
number_of_years = end_date - start_date
# Step 1
date_filter = data_daily
initial_investment = 10000
monthly_deposit = 1000
# Start Price
start_adjusted_close_price = date_filter['5. adjusted close'][-1]
starting_shares = initial_investment / start_adjusted_close_price
start_reported_date = date_filter['date'][-1] #not working
# End Price
end_adjusted_close_price = date_filter['5. adjusted close'][0]
end_reported_date = date_filter['date'][0] #not working
# Final Math
step1_final_value = round((starting_shares * end_adjusted_close_price), 2)
step1_profit = round((step1_final_value - initial_investment), 2)
step1_roi = round((((step1_final_value - initial_investment) / initial_investment)* 100), 2)
step1_cagr = round((((math.log(step1_final_value/initial_investment)) / (math.log(1 + number_of_years)))* 100), 2)
# Print out results
print("Starting Date : {} ".format(start_reported_date))
print("Start Share Price : ${} ".format(start_adjusted_close_price))
print("Starting Shares : {} ".format(starting_shares))
print("")
print("Ending Date : {} ".format(start_reported_date))
print("Ending Share Price : ${} ".format(end_adjusted_close_price))
print("")
print("Total Deposits : ${} ".format(initial_investment))
print("Portfolio Value : ${} ".format(step1_final_value))
print("Investment Profit : ${} ".format(step1_profit))
print("ROI : {}% ".format(step1_roi))
print("CAGR : {}% ".format(step1_cagr))
I have tried this one that was most recommended and a few others
data_daily.loc[datetime.date(year=2014,month=1,day=1):datetime.date(year=2015,month=2,day=1)]
But I keep getting empty dataframe
Empty DataFrame
Columns: [1. open, 2. high, 3. low, 4. close, 5. adjusted close, 6. volume, 7. dividend amount, 8. split coefficient]
Index: []
Any help is much appreciated! :)