Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial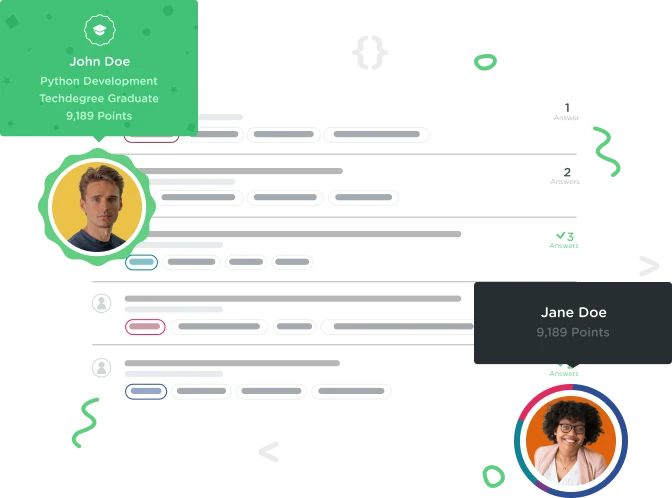
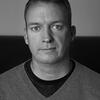
Andy Hughes
8,479 PointsHow to modify this for updating things
I keep writing this and ending up with pages, so I'll bullet point it to be concise :)
1) Trying to create an update function for the social app. 2) Can't get it to update the database with the new information. Info just stays the same. 3) Tried .save() and .update() but neither makes a difference.
I'm building on what Kenneth developed so it's Sqlite, Flask, Peewee.
What am I trying to achieve? 1) I have a view that shows a database table. Each row has a 'delete' and 'update' link. 2) Clicking 'update' uses the ID from the row to take the user to a form that should allow them to update that row. 3) The form seems to work ok and I get no errors, but the database functionality is not updating the row and that's what I want it to do.
I need some help :) Relevant code is below.
app.py
'''updates a standard'''
@app.route('/update_standard/<int:id>', methods=('GET', 'POST'))
@login_required
def update_standard(id):
form = forms.UpdateForm()
if form.validate_on_submit():
models.Standards.change_standard(
section=form.section.data,
standard=form.standard.data)
flash("Standard updated! Thanks!", "success")
return redirect(url_for('view_standards'))
return render_template('update-standard.html', form=form, id=id)
models.py
class Standards(Model):
section = CharField(unique=False)
standard = CharField(unique=True)
class Meta:
database = DATABASE
@classmethod
def create_standard(cls, section, standard):
try:
with DATABASE.transaction():
cls.create(
section=section,
standard=standard
)
except IntegrityError:
raise ValueError("Section or Standard already exists")
@classmethod
def delete_standard(cls, id, section, standard):
try:
with DATABASE.transaction():
cls.delete(
id=id,
section=section,
standard=standard)
except IntegrityError:
raise ValueError("Standard does not exist for deletion")
@classmethod
def change_standard(cls, section, standard):
try:
with DATABASE.transaction():
cls.update(
section=section,
standard=standard)
except IntegrityError:
raise ValueError("Standard does not exist for update")
forms.py
class UpdateForm(FlaskForm):
section = TextAreaField('Enter new section', validators=[DataRequired(), section_exists])
standard = TextAreaField('Enter new standard', validators=[DataRequired(), standard_exists])
1 Answer

jb30
44,806 PointsIn models.py
, try changing change_standard
to
@classmethod
def change_standard(cls, id, section, standard):
try:
with DATABASE.transaction():
standards_to_update = cls.get_by_id(id) # specifies which Standards to update
standards_to_update.section = section # updates the value for section
standards_to_update.standard = standard # updates the value for standard
standards_to_update.save() # saves changes
except DoesNotExist:
raise ValueError("Standard does not exist for update")
and passing in id
as an argument for models.Standards.change_standard
in app.py
models.Standards.change_standard(
id=id,
section=form.section.data,
standard=form.standard.data)
Andy Hughes
8,479 PointsAndy Hughes
8,479 Pointsjb30 - Sir, you are a bloomin superstar! Thank you. It works perfectly.
Just let me work through the logic to see if I understand what you're answer is doing. :)
1) change_standard is receiving the ID, Section, Standard from my form view? 2) you then look for the passed in record with the 'get_by_id(id)'? 3) you then relate 'section' and 'standard' to the 'standards_to_update' variable that already knows the record from the ID? 4) You then use the save() to update the database?
It's just important for me to understand how it works in case I need to do it again. :)
Thank you again for the support.
jb30
44,806 Pointsjb30
44,806 Points1)
change_standard
is receiving the section and standard from the form, and the id fromdef update_standard(id):
2) Yes. There are ways to find which record it is from the section or standard, but you might be changing those values in
change_standard
and the section and standard are not guaranteed to be unique.3) Yes.
4) Yes.