Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial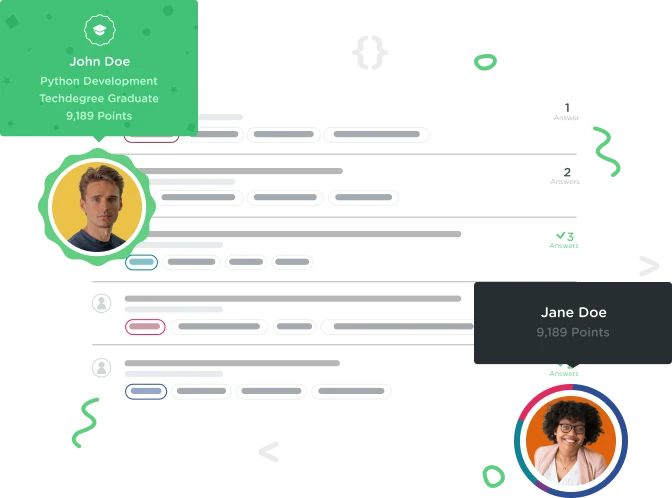

Adam maitland
Courses Plus Student 211 PointsHow to send info from a form to a database with PDO
How to send info from a form to a database with PDO ?
<?php
if($_SERVER['REQUEST_METHOD'] == 'POST') { // testing if form is post
$userName = $_POST['name'];
$userPassword = $_POST['password'];
$servername = 'localhost';
$username = 'root';
$password = 'root';
try {
//conects to the database
$db = new PDO("mysql:host=$servername;dbname=Test", $username, $password);
/*** echo a message saying we have connected ***/
echo 'Connected to database <br><br><br>';
// adds info to the database
$db->exec("INSERT INTO `Test`.`users` (`id`, `user_name`, `password`) VALUES (NULL, $userName, $userPassword)");
}catch(PDOException $e) {
echo "not conected<br>";
}
}
?>
1 Answer
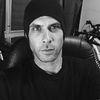
jack AM
2,618 PointsHi Adam,
So I am not sure exactly, what code you have in what files, or if you have all of this php in one file. But going off of what you wrote, as I understand sometimes you just want something to get going, here is something that works, that will query a database and check if the username and password match. This is all in one index.php file (not recommended)...
<?php
/*
* index.php
*/
if (isset($_POST['login'])) {
$formMessage;
$query;
$username = $_POST['username'];
$password = $_POST['password'];
$table = "User";
$server = 'localhost';
$database = 'Test';
$dbUsername = 'admin_dba';
$dbPassword = '.(Admin).';
try {
$pdo = new PDO("mysql:host=". $server. ";dbname=". $database, $dbUsername, $dbPassword);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$query = $pdo->prepare("SELECT * FROM ". $table. " WHERE username = :username AND password = :password");
$query->bindParam(":username", $username);
$query->bindParam(":password", $password);
$query->execute();
$user = $query->fetch(PDO::FETCH_ASSOC);
if ($user) {
$formMessage = "Welcome ". $user['username']. "!";
} else {
$formMessage = "Username or Password incorrect";
}
} catch(PDOException $e) {
$formMessage = "ERROR : ". $e->getMessage();
}
}
?>
And then lower down in the body tag
<form action="<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>" method="post">
<input name="username" placeholder="Username" type="text"></input>
<input name="password" placeholder="Password" type="password"></input>
<button name="login" type="submit">Submit</button>
<div><?php if (isset($formMessage)) { echo $formMessage; } ?></div>
</form>
I hope this gets you're immediate flow going, but what I would recommend, is to take a look at using pdo prepared statements as to prevent SQL injection. Take a look here http://php.net/manual/en/pdo.prepared-statements.php. In the example given, it also binds parameters to variables in the prepared statement, which is really cool. Take a look at bindParam here http://php.net/manual/en/pdostatement.bindparam.php
Lastly, I noticed in your code, you used the two variable names, $username, and $userName. Be very wary of utilizing the same variable name like that, if you notice I used $dbUsername, and $username (again just as an example). It will save you alot of time debugging, if variable names are distinguishable. But if you'd like for me to touch on anything else/more, feel free and lemme know, happy coding!