Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial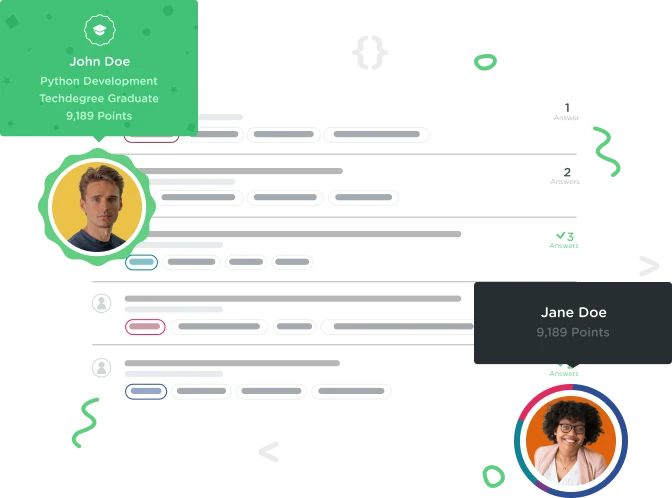
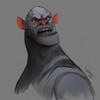
Filipe Strazzeri
536 PointsHow would you go about catching the overflow exception Jeremy talks about in the end of the video ?
Hi all, thanks for taking a look at this !
I am curious if you have to add another Try or just another Catch inside the same class ?
For example here is my code:
catch(FormatException)
{
Console.WriteLine("Please use numbers or \"quit\" for the program to work");
continue;
}
Would this be correct to just add at the end of my code?
catch(FormatException)
{
Console.WriteLine("Please use numbers or \"quit\" for the program to work");
continue;
}
catch(OverflowException)
{
Console.WriteLine("Please use numbers or \"quit\" for the program to work");
continue;
}
Not sure what kind of numbers I would have to get to trigger the exception but when I spam a bunch of 9's the console returns this number : 1E+72 , I am no good at math so maybe the overflow is just very very very large? Maybe don't worry about triggering it ?
Thanks!
2 Answers

andren
28,558 PointsYou don't need multiple try/catch blocks, your guess that you can define multiple catch blocks for a single try block is correct. The code you show in your example is completely valid.
The only thing you need to be aware of is that is that the catch blocks has to be sorted in such a way that one catch block won't catch an exception that a catch block below it was meant to catch. If you use a catch block to catch exceptions of type Exception
for example (which is the exception class all exceptions inherit from) then that block would catch all exceptions. If you placed that at the top then any more specific catch blocks down below would never execute since the first catch block would always catch the exception.
The C# compiler will actually refuse to compile your code in such a situation. If you place the Exception
catch block as the last one though then it won't be a problem, since the more specific catch blocks will end up catching the exception first.
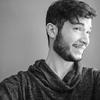
Damian Adams
21,351 PointsIf you want to catch an OverflowException
for an arithmetic operation you need to use the checked
keyword on the block of code that you expect the exception to be raised. Then you can attempt to catch it. This is necessary because the compiler does not perform overflow checks on arithmetic by default and insteads wraps the operation around the minimum or maximum value of the type.
As Microsoft's own C# documentation states:
For the arithmetic, casting, or conversion operation to throw an OverflowException, the operation must occur in a checked context. By default, arithmetic operations and overflows in Visual Basic are checked; in C#, they are not. If the operation occurs in an unchecked context, the result is truncated by discarding any high-order bits that do not fit into the destination type.
See the Remarks section on this page of the MSDN: https://msdn.microsoft.com/en-us/library/system.overflowexception(v=vs.110).aspx
Filipe Strazzeri
536 PointsFilipe Strazzeri
536 PointsThanks Andren ! I am glad my guess was correct and will look out for the issue you mentioned as well !
Cheers!