Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial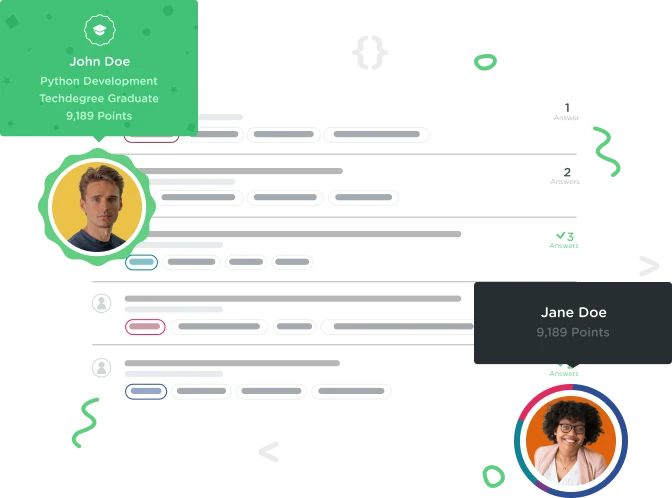

James Miller
403 PointsI am trying to write an if statement that will produce console output if firstExample = secondExample
String firstExample = ("hello"); if (firstExample.equals("%s", secondExample)) { console.printf("first is equal to second"); } String secondExample = ("hello"); String thirdExample = "HELLO";
What am I doing wrong I have tried this a dozen ways but I can't seem to get it
// I have imported a java.io.Console for you, it is named console.
String firstExample = ("hello");
if (firstExample.equals("%s", secondExample)) {
console.printf("first is equal to second");
}
String secondExample = ("hello");
String thirdExample = "HELLO";
8 Answers
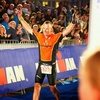
Steve Hunter
57,712 PointsWe start with this:
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
The question is, Add an if statement that checks to see if firstExample is equal to secondExample. If it is, print out "first is equal to second".
So, after we have declared and initialized/assigned the three string variables, we need to use an if
statement to see whether firstExample
equals secondExample
. As in the note above, this requires us to use the equals
method on the String object. (It works on many/most objects too as the equals
method comes from the superclass, Object
. I digress). Key here, is the emphasis on after. You can't use a variable until it has been declared and assigned, so any usage of variables must happen in a line subsequent to the one it is created in.
We call methods on objects using dot notation. Methods take their parameters inside brackets after the method name. So, doesThis.equals(thisObject)
, for example. Using our context, the equality comparison would look like:
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if(firstExample.equals(secondExample)){
console.printf("first is equal to second");
}
You have confused yourself with the %s
formatter notation. This is about strings, yes, but is used to interpolate them into other strings. You don't need %s
except when you are outputting strings. Let me make up another lame example:
// read in a user's name
String myName = console.readLine("What's your name?: ");
// output a users name
console.printf("Thanks, %s. Nice to meet you", myName);
So, here we use %s
to mark the point in the output string where we want the contents of myName
inserting. That outputs "Thanks, Steve. Nice to meet you
".
Make sense? Shout back, if not - and sorry for the delayed response; I was out for a curry! Yum
Steve.
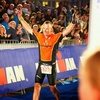
Steve Hunter
57,712 PointsHi there,
Just one point on the above answer; we shouldn't really use ==
to check for string equality.
The reasons are covered in Craig Dennis' course, The Thing About Strings and are also explained better than I'm able to in this post on S.O.. There's another TH thread here too.
While you can probably get through a challenge using ==
, it is better to use the .equals()
method to check for logical equality, rather than testing for the two things being the same in memory.
It's a small point, but one worth making as it is certainly best practice. The course video covers the equals()
method just before the 2 minute point.
Steve.

Andrew Winkler
37,739 PointsFirst off, why are you surrounding the strings in parentheses? Doing so is unnecessary.
Second, it's best practice to define your variables before running any functions that use those variables - that way your functions actually have something to work on.
Third, clean code is easier to recognize patterns within and learn from. I would encourage you to benefit, doing so in the future.
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample == secondExample) {
console.printf("first is equal to second");
}
Also, I wrote this from my phone :D

James Miller
403 PointsGents I really appreciate the responses. Unfortunately none of these are pointing out the error in my code. I have tried it with and with out parenthesis. I need to know why this, if (firstExample.equals("%s", secondExample)) equals. method is not functioning.
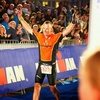
Steve Hunter
57,712 PointsHi there,
Try this:
if(firstExample.equals(secondExample)){...
Steve.
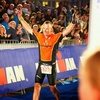
Steve Hunter
57,712 PointsDo the rest the same you have...

Andrew Winkler
37,739 Points+1 to Steve. I was tired when I wrote my answer.
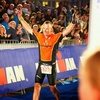
Steve Hunter
57,712 Points
James Miller
403 PointsJavaTester.java:106: error: cannot find symbol if (firstExample.equals(secondExample)) { ^ symbol: variable secondExample location: class JavaTester 1 error

James Miller
403 PointsI have tried this every way I can imagine. But doesn't %s need to be used to mark the String for secondExample?
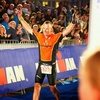
Steve Hunter
57,712 PointsIn short; no.
I'll walk through the challenge - give me a minute ...
James Miller
403 PointsJames Miller
403 PointsI drove myself crazy because I wrote it before the declared variables lol. Thank you guys so much. This is how i wrote it the first time. Just wrote it underneath the first string.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYup. If ya ain't made it, ya can't use it!
Shout if you need any help in the future. Here to help.
Steve.