Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial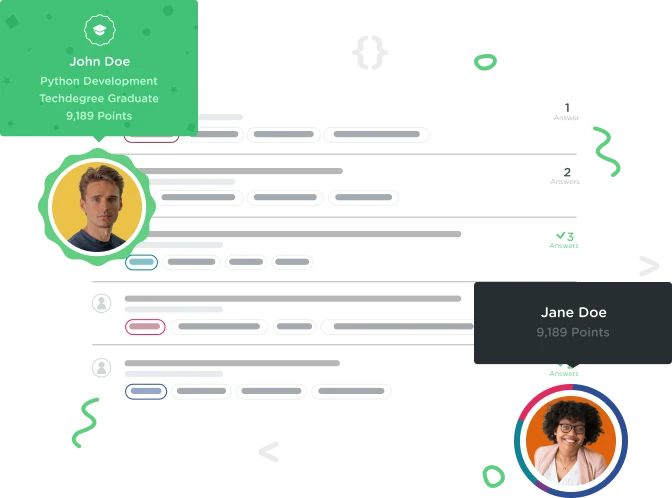
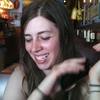
Holly Lewis
3,574 PointsI cannot figure out what I am doing wrong here on Challenge to fix getLineNumber.
I have probably just over complicated it. I know the code below has errors, I just have tried about 30 different ways and I cant figure out what is going on. Im getting so frustrated. Any help would be appreciated, Id like to move forward
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
String lastName = lastName.charAt(0);
if ('M' <= 'M'){
int lineNumber == 1;
} else {
int lineNumber == 2;
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers
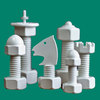
Steven Parker
241,970 PointsHere's a few hints:
- a variable should only be defined once, so references to "lineNumber" should not have "int" in front
- "lastName" is already defined as a String argument, use a different name for a new variable
- the variable to hold the result of a "charAt" should be Character type
- the comparison "
'M' <= 'M'
" will always be true, you probably meant to have a variable on one side - the assignment operator is "=", a "==" is a comparison operator
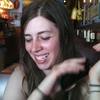
Holly Lewis
3,574 PointsI got it!
if ( lastName.charAt(0) <= 'M' ) { lineNumber = 1; } else { lineNumber = 2; }
Holly Lewis
3,574 PointsHolly Lewis
3,574 PointsThank you for the help. I knew I was just going down a code rabbit hole! Over complicating, as usual. I started over and got much closer but I am not sure how to do the else correctly. I have never seen something passed into it because as far I know thats not how else works. So I have been messing with it but basically my line #1 seems to be catching everything and if I change the <,>,= then it just pulls everything into line 2. You did help alot but can you tell what I am doing wrong here?
Here is what I have now :
public int getLineNumberFor(String lastName) { int lineNumber = 0; lastName.charAt(0);
}