Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial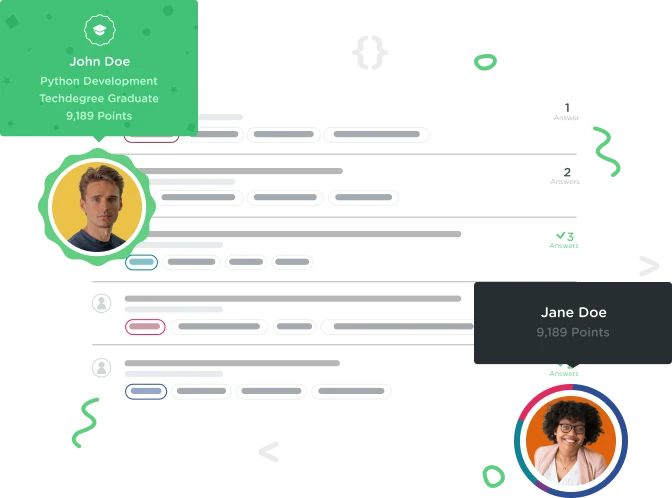
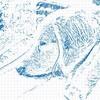
oliverchou
20,886 PointsI can't meet the challenge!
Here's my code, and I just can't pass the callenge! What does it want me to do?
<?php
//edit this array
$contacts[] = array(
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
);
$contacts[] = array(
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
);
$contacts[] = array(
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
);
$contacts[] = array(
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$contacts[0]['name'] : $contacts[0]['email']</li>\n";
echo "<li>$contacts[1]['name'] : $contacts[1]['email']</li>\n";
echo "<li>$contacts[2]['name'] : $contacts[2]['email']</li>\n";
echo "<li>$contacts[3]['name'] : $contacts[3]['email']</li>\n";
echo "</ul>\n";
2 Answers
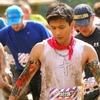
egaratkaoroprat
16,630 PointsHi oliverchou
The challenge was very hard to understand in my opinion... But after playing around I got the final solution (you can figure out which one belongs to which objective :)).
It probably failed because of the output, as there was an issue converting the array to string.
<?php
//edit this array
$contacts = array(
array('name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'),
array('name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'),
array('name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'),
array('name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com')
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>" . $contacts[0]['name'] . " : " . $contacts[0]['email'] . "</li>\n";
echo "<li>" . $contacts[1]['name'] . " : " . $contacts[1]['email'] . "</li>\n";
echo "<li>" . $contacts[2]['name'] . " : " . $contacts[2]['email'] . "</li>\n";
echo "<li>" . $contacts[3]['name'] . " : " . $contacts[3]['email'] . "</li>\n";
echo "</ul>\n";

bhateam
8,765 PointsI solved this by concatenating the Associative Array to the echo like this.
echo "<li>". $contacts[0]['name'] . " : " . $contacts[0]['email'] . "</li>\n";
However when I was looking for a way to explain why you have to concatenate the associative array. I found out that you can put curly braces {} around the associative array and it will interrupt the value of the associate array.
echo "<li> {$contacts[0]['name']} : {$contacts[0]['email']} .</li>\n";
This blog post explains it better than I can. I am still learning php too.