Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial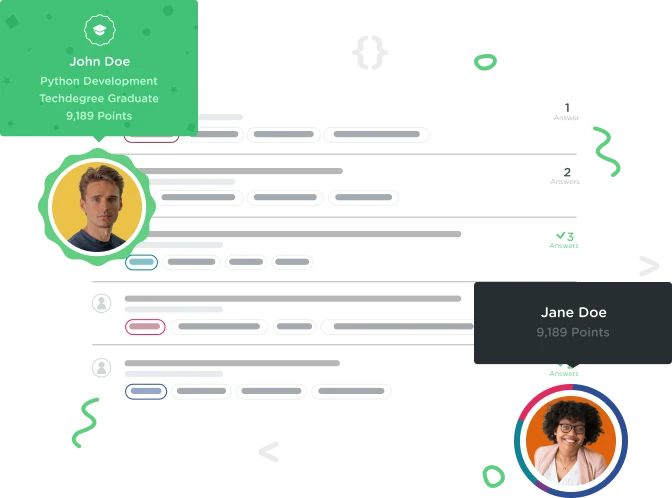

Abdullah Jassim
4,551 PointsI didnt understand this video from 3:50 onwards. The following are my specific questions:
1) How does the .pop() function change the list?
- my understanding of the .pop() function is that it merely returns either the last item or the indexed item. So how does this change the list?
2) I did not understand the explanation of how it changes in 4:36 - 4:48.
- My understanding is that in def DISPLAY_WISHLIST, both DISPLAY_NAME and WISHES are parameters and they are objects. The labels applied to these parameters are Books and books. Correct? After this I still dont understand how .pop changes the list item.
3) How does making a copy of the list not change the list?
Thanks in advance.
2 Answers
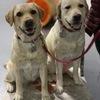
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Abdullah,
1) Your understanding of pop()
is not quite correct. Let's look at the documentation:
list.pop(i) Remove the item at the given position in the list, and return it.
It is the removing the item from the given position part that modifies the list.
2) Yes, display_name
and wishes
are parameters, and they are objects (everything in Python is an object). "Books"
and books
are the arguments provided for those parameters. The reason that pop()
changes the list item is entirely down to the behaviour of the pop
method itself, which always changes the list on which the method is called.
Example:
my_list = ["hello", "there", "abdullah"]
word = my_list.pop(0)
print(word) # hello
print(my_list) # ['there', 'abdullah']
As you can see in this simple example, pop()
always does two things: 1: it takes the specified item (or last item if None specified) out of the list and 2: it returns that item.
3) Making a copy of the list is creating an entirely new list and filling it with the items that are in the old list. Consider this simple example:
my_list = ["hello", "there", "abdullah"]
word = my_list[0]
print(word) # hello
print(my_list) # ['hello', 'there', 'abdullah']
Hopefully it's clear that just reading a value from a list and putting that value in a variable doesn't modify the original list. We can extend the example slightly:
my_list = ["hello", "there", "abdullah"]
words = my_list[0:2]
print(words) # ['hello', 'there']
print(my_list) # ['hello', 'there', 'abdullah']
Here we've done basically the same thing, but instead of reading one value out of the list, we've read the items from index 0 ('hello') to the last item before index 2 ('there'). Again, we're just reading the values out of the original list, so the original list remains unchanged.
Lastly, we can extend the example to copying the whole list:
my_list = ["hello", "there", "abdullah"]
words = my_list[:]
print(words) # ['hello', 'there', 'abdullah']
print(my_list) # ['hello', 'there', 'abdullah']
As you can see, this is exactly the same, except we are telling Python to read all the values from the beginning of the original list to the end of it.
Hope that clears everything up for you
Cheers
Alex

Derek Lefler
2,608 Pointsdef display_wishlist(display_name, wishes):
print(display_name + ":")
suggested_gift = wishes.pop(0)
print("======>", suggested_gift, "<=======")
for item in items:
print("* " + item)
print()
.pop() returns an item from the list and then drops off that item from the list (the last item if not specified). So in the lesson's example,
suggested_gift = wishes.pop(0)
print("======>", suggested_gift, "<=======")
pop(0) returned wishes and then removed the first item from the list.
for wish in wishes:
print("* " + wishes)
So when this part of the function is executed, wishes doesn't include the book "Learning Python..." or the video game "Legend of Zelda...." because pop(0) removed it before.
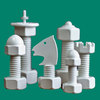
Steven Parker
231,248 PointsFYI: "pop" removes the last item if not specified.

Derek Lefler
2,608 PointsSteven Parker My mistake, you're correct.

Abdullah Jassim
4,551 PointsGot it now. Thanks for the explanation.
Abdullah Jassim
4,551 PointsAbdullah Jassim
4,551 PointsThanks for that detailed explanation. Quick question - in the above example, isnt 2 - 'abdullah'? How come its only capturing till 'there'?