Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial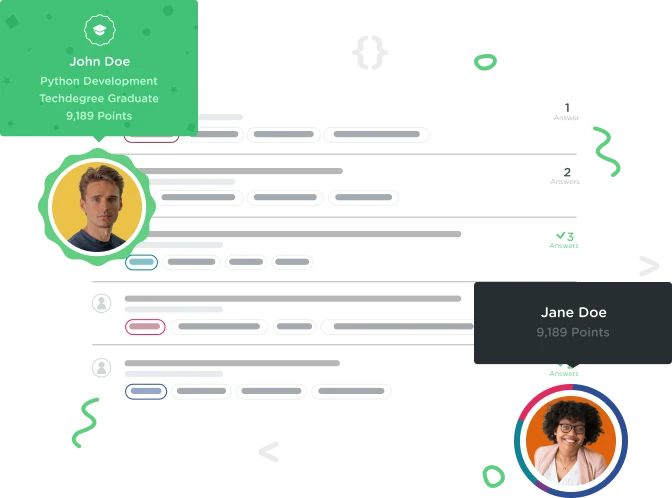

Jiho Song
16,469 PointsI don't have a clue
How shall I begin with? i don't seem to have a clue regarding this task.
I have been watching previous lecture a few times but still the same:(
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter)
{
return 0;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer
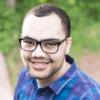
Philip Gales
15,193 PointsNow in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method. Make sure to check Example.java for some example uses.
In other words, you passed in a char (letter) and want to see how many times that letter is in tiles. Say for example I have the following tiles ("hdynbeejk"). I can then ask my getCountOfLetter() method how many times the letter "e" is in tiles and it will return 2.
If I did this in real life, I would take the letter and compare it to each letter in tiles. More specifically, I would compare my char (letter) to each char in the String (tiles). Remember, Strings are an array of Chars.
If you want to try this on your own, stop reading at this point.
To get started, I would use a for loop to loop through each of the tiles.
public int getCountOfLetter(char letter) {
for (char tile : tiles.toCharArray()) {
}
}
Notice I used a for each loop. That is, it will loop through each char in tiles and will use tile to refer to the specific char in tiles.
I would then see if my letter matches the tile.
public int getCountOfLetter(char letter) {
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
}
}
}
If they do match, I would add 1 to my count. So let's create a counter.
public int getCountOfLetter(char letter) {
int count = 0;
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
count++;
}
}
}
Finally, we need to return the number of matches, or count.
public int getCountOfLetter(char letter) {
int count = 0;
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
count++;
}
}
return count;
}
Hope this helped explain the logic behind the answer. Thinking out a challenge and writing down the steps to how you would solve it in real life, before you begin to code, will often make a challenge easy to solve.
Jiho Song
16,469 PointsJiho Song
16,469 PointsThanks but what that notion : mean? I havent heard of that thus far. And toCharArray as well... May I should start studying with some java textbook for a novice? I find little difficult to begin with mere treehouse
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsI think both were taught in the java basics course. I wouldn't worry about it though and just programming.
The best thing you can do at this point is to make your own console apps outside of treehouse.
Abdul Jamac
1,049 PointsAbdul Jamac
1,049 PointsBest explanation Ive seen , thank you.
Elias Svoba
14,347 PointsElias Svoba
14,347 Pointsi followed your steps but i'm getting an error message on the compiler directing me to this part of the code [ for(char tile : tiles.CharArray()) ] .
cannot find symbol for(char tile : tiles.CharArray()) symbol: method CharArray()