Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial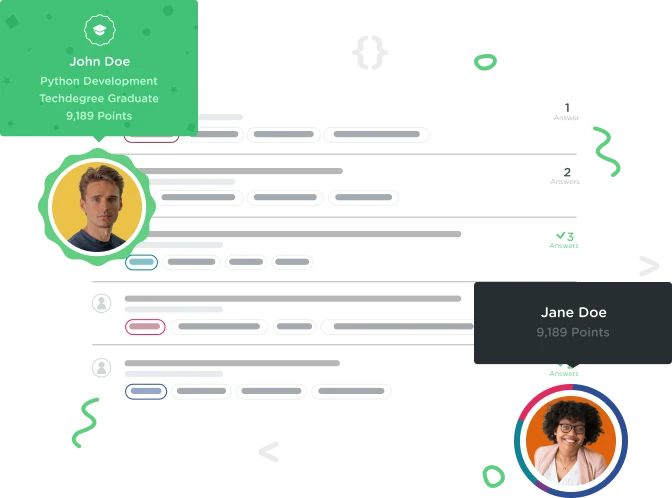

Travis Williams
2,049 PointsI don't understand
It says to initialize the constructor with ToungeLength which I did and its not working.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int TongueLength)
{
TongueLength = TongueLength;
}
}
}
1 Answer
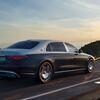
Balazs Peak
46,160 PointsYou are referencing two different things with the same variable name, which causes ambiguity.
C# has a built in feature to handle these situations. If the class variable and the local variable passed in as a parameter would have a different name, you wouldn't need to specify which one are you trying to influence. But in this case, you named both the same. In this case:
- with "TongueLength", you can refer to the local variable of the constructor function, passed in as a parameter.
- with "this.TongueLength" , you can refer to the class variable. "This" keyword is a reference to the current object instance, which is just being created by the constructor.
public Frog(int TongueLength)
{
this.TongueLength = TongueLength;
}
Another solution would be using another name for the parameter to avoid ambiguity.
(Please note that this is not considered best practice among C# developers. Using the "this" keyword is more common solution. )
public Frog(int tongueLengthParameter)
{
TongueLength = tongueLengthParameter;
}
It is also worth mentioning, that you can use the this keyword in the latter case as well, but in that case, it is not necessary to avoid ambiguity.
public Frog(int tongueLengthParameter)
{
this.TongueLength = tongueLengthParameter; // you can use "this" keyword, even when it is not necessary to avoid ambiguity
}