Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial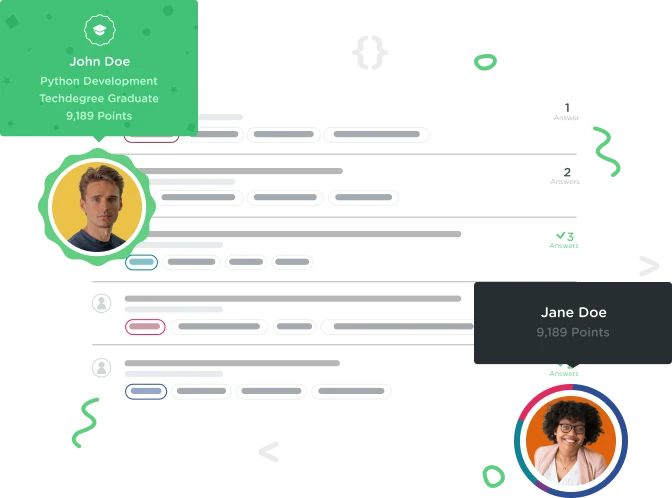
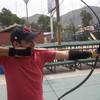
Beto Najera
7,072 PointsI don't understand how to solve this and i've tried many ways.
I have some problems with this challenge.
<?php
//edit this array
$contact1 = array('Alena Holligan' => 'alena.holligan@teamtreehouse.com');
$contact2 = array('Dave McFarland' => 'dave.mcfarland@teamtreehouse.com');
$contact3 = array('Treasure Porth' => 'treasure.porth@teamtreehouse.com');
$contact4 = array('Andrew Chalkley' => 'andrew.chalkley@teamtreehouse.com');
$contacts = array($contact1, $contact2, $contact3, $contact4);
var_dump($contacts);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
2 Answers
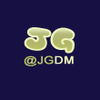
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi there Beto,
The echos that you;ll use to display the data have been provided for you, as well as the values. What you have to do is find a way to display the values dynamically rather than just as hard coded strings.
This is simple enough to do. HINT.
- You need to display the values by their key `
e.g. $array["value"};
- You'll also need to concatenate the strings as if you try to includes the values as part of a single string it won't work.
- Have a look at the strings in the echo and where these match up with the values in the
$contacts
array.
Good luck!
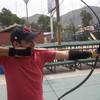
Beto Najera
7,072 PointsThanks you both for your help. I can't solve that yet but I'll keep in mind what you say
Ryan Breece
4,160 PointsRyan Breece
4,160 PointsInstead of adding 4 separate $contact arrays, you need to add separate arrays into the same contact array using 'name' as the key.
then add the email as the key
finally, concatenate the variables with the strings in the echo