Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial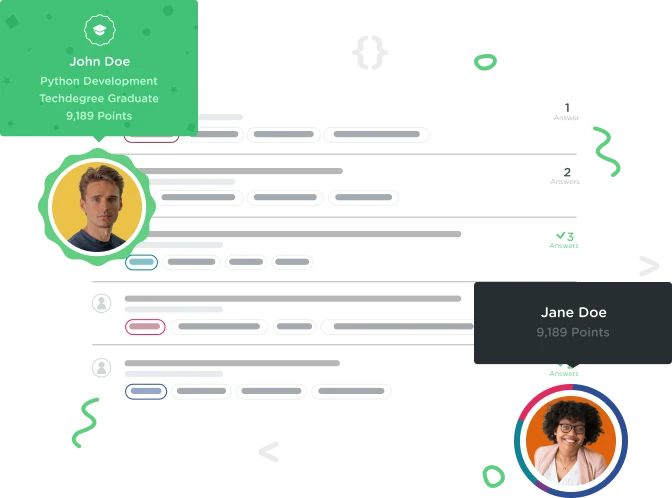
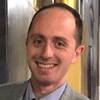
Clifford Gagliardo
Courses Plus Student 15,069 PointsI don't understand this problem or how this solution fits the bill. This challenge seemed to come out of left field.
Where did this question come from? I feel like I got tricked into a sucker punch.
I don't understand how this solution works. I still feel like I have a poor understanding of class methods and why and how to use them. Basically, after inheritance, I feel like my comprehension went way downhill. The teachers notes don't seem to have anything that reinforces what the video talked about, so I'm searching around the transcript for information that is not presented in a manner that fits the code. I really would like better teachers notes, like maybe "handouts" or something.
from dice import D20
class Hand(list):
def __init__(self, size=0, die_class=D20):
self.size=size
super().__init__()
for _ in range(size):
self.append(die_class())
@classmethod
def roll(cls, size=2):
return cls(size)
@property
def total(self):
return sum(self)
class Hand(list):
@property
def total(self):
return sum(self)
2 Answers

Josh Keenan
20,315 PointsSo, why this question? To test you and stretch you a bit. A lot of the challenges can be a bit much when you first start them and I think they're designed to be that way, not easy to solve at all and to really make you think about it.
Why use methods?
Methods are functions that only a specific class can use. You have seen them since the start of studying Python and they're really powerful, '''python List.append()''' is one, in fact anything that uses dot notation is accessing something from a class somewhere. Methods let you define functions for certain objects only, so that only the class and any that inherit from it can use them.
How to use them?
Well let's say we have a dice class that has a roll method, '''python dice.roll()''' runs that method for you.
How to not feel as lost?
Practice. Then practice some more. Then go back and watch the videos and do all the challenges again, it'll really help you out when it comes to learning the technology.

Kimmo Ojala
Python Web Development Techdegree Student 8,257 Pointsfrom dice import D20
Class Hand takes in a class type "die_class" which is set to D20 and a variable called size. It passes nothing to its superclass "list". It appends to itself (being of type list) objects that are created by calling die_class() as many times as there are items in "range(size)". Check https://teamtreehouse.com/library/giving-a-hand for another example.
class Hand(list): def init(self, size, die_class=D20): super().init() for _ in range(size): self.append(die_class())
This is a property of the class Hand which is called like an attribute of a Hand instance (object). E.g. >>> h = Hand(2) >>> h.total. Sum is a built-in function of Python which sums the items of an iterable. Objects belonging to class Hand are iterables because the superclass of class Hand is "list". Objects of class D20 within class Hand can be added to another (summed) because their superclass is class Die, which contains an add method.
@property
def total(self):
return sum(self)
This is a class method within class Hand. The class method creates a new instance of class Hand as it passes the variable size on to the constructor of the class Hand (def init (self, blah, blah)). The class method is called like any other method, but instead of an intance of class, the class itself is referred. E.g. >>> h = Hand.roll(2). In this example h is a new instance of class Hand, which is created by calling the class method "roll".
Check https://teamtreehouse.com/library/constructicons for another example.
@classmethod
def roll(cls, size):
return cls(size)