Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial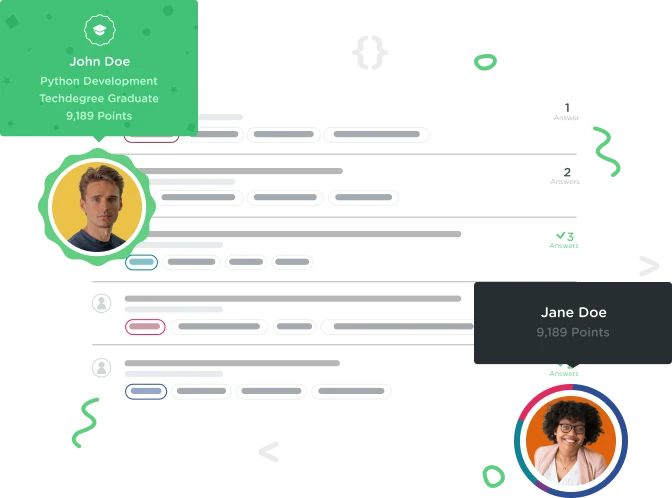
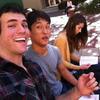
Joshua Jones
4,756 PointsI don't understand what this question is asking me to code.
What exactly does it want me to implement?
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides=20)
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls):
x = Hand()
x.append(D20())
x.append(D20())
return x
1 Answer

Jon Mirow
9,864 PointsHi there!
Ah don't worry I got stuck here for ages too!
In this instance, the problem is that your code is too tightly bound to the example in the question. You're spot on that Hand.roll() needs to be a @classmethod (kudos!), but it also needs to be able to take an argument, and they will test values other than 2 (it says I'm going to use code similar to Hand.roll(2)).
At present if you called Hand.roll(2), you'd get
TypeError: roll() takes 1 positional argument but 2 were given
All you need to do is modify your Hand.roll so it can take a numerical argument and return a Hand instance containging that number of D20 elements. For example:
>>>Hand.roll(2)
[<D20 object at 0x7f968b443208>, <D20 object at 0x7f968b443240>]
>>>Hand.roll(0)
[]
>>>Hand.roll(4)
[<D20 object at 0x7fcf3f725278>, <D20 object at 0x7fcf3f7252b0>, <D20 object at 0x7fcf3f7252e8>, <D20 object at 0x7fcf3f725320>]
Maybe the roll method could be simplified and the Hand class made more usable by seperating some of the logic into a Hand.__init
__ method as well?
A quick tip: In this example, you'll have to add an import line to your hand module so it knows about your D20 class, and remember in a @classmethod, you don't have to specify the class - cls() and Hand() are identical here.
Hope it helps!