Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial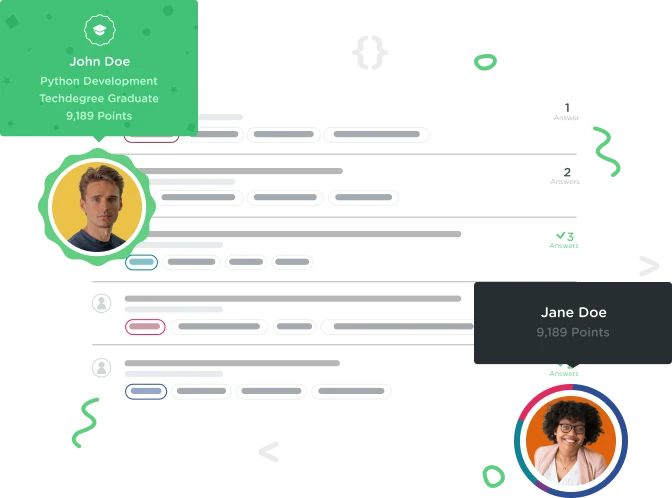

Christopher Blanton
19,337 PointsI get the error message: `Didn't get 'D20's in my 'Hand' instance` when appending D20s to the hand.
When I put this into my interpreter it gives me a list of D20s and I am definitely appending D20s to the dih list? I know I'm missing something and I bet it's going to be silly.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides=20)
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, qty):
dih = []
for _ in range(qty):
dih.append(D20)
return dih
2 Answers
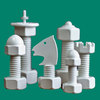
Steven Parker
231,248 PointsIt looks like the D20's are being added to an ordinary list instead of an instance of "Hand". The "cls" function is useful for creating a new Hand.

Christopher Blanton
19,337 PointsI get this error when passing the list to the class as well using cls(dih).
Steven Parker
231,248 PointsSteven Parker
231,248 PointsOh, and you also need parentheses when you create a D20 instance:
dih.append(D20())
Christopher Blanton
19,337 PointsChristopher Blanton
19,337 PointsUsing the parenthasis after the D20 was the issue, thanks!