Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial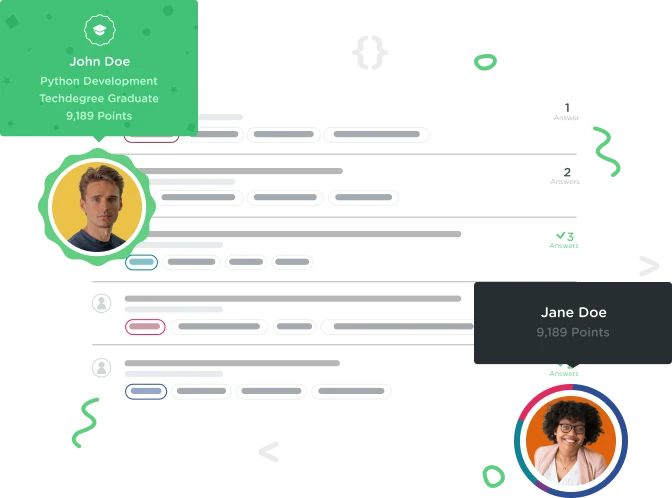

Fatemeh Saryazdi
5,369 PointsI have difficulty with getting my code compiled for Polygon and Square class. Can you please provide the solution?
Seems like the sub-class Square has the attribute "SideLength" that the base class doesn't have that. How I can use constructor in this case?
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength
public Square (int NumSides, int sideLength): base (4)
{
SideLength = sideLength;
}
}
}
1 Answer

andren
28,558 PointsThe are two issues with your code:
- You have forgotten a semicolon after the
sideLength
declaration. - The constructor for the Square class does not need to have a parameter for the number of sides. A Square will always have 4 sides (that's a pretty fundamental part of being a square) so having to pass it in as an argument is pointless.
If you fix those two issues like this:
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square (int sideLength): base (4)
{
SideLength = sideLength;
}
}
}
Then your code will work.
The fact that the Square has an attribute that the Polygon does not have is not really an issue, as the constructor of an inherited class is independant of its parent constructor in terms of what parameter you do or do not include. The only requirement is that you initialize the parent class by passing in arguments to its constructor in the base
call. Which you are doing correctly in your code.
Fatemeh Saryazdi
5,369 PointsFatemeh Saryazdi
5,369 PointsThanks Andren. I figured it our soon after posting the discussion. Thanks anyways!