Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial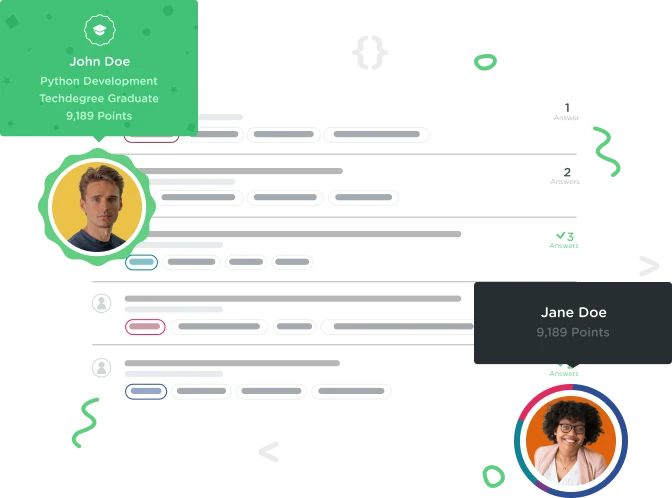

Dennis Boccardi
1,099 PointsI have no idea what to do. Multi-dimensional arrays.
What am I suppose to title each array?
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";

Dennis Boccardi
1,099 PointsOn the second task though, it says,
Add a second element with the key 'email' to the internal array of each person. You'll find the correct email for each person in the hard coded output.
And my code looks correct
<?php //edit this array $contacts = array ( $contacts[] = [ 'name' => 'Alena Holligan' 'email' => 'alena.holligan@teamtreehouse.com' ], $contacts[] = [ 'name' => 'Dave McFarland' 'email' => 'dave.mcfarland@teamtreehouse.com' ], $contacts[] = [ 'name' => 'Treasure Porth' 'email' => 'treasure.porth@teamtreehouse.com' ], $contacts[] = [ 'name' => 'Andrew Chalkley' 'email' => 'andrew.chalkley@teamtreehouse.com' ] );
But, it keeps saying,
Oops! It looks like Task 1 is no longer passing.
3 Answers
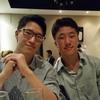
David Choi
8,536 PointsIt seems like you are adding elements to the array $contacts, while you are also declaring it. Furthermore, your syntax for adding elements to the array is wrong.
This is your code:
$contacts[] = [ 'name' => 'Alena Holligan' 'email' => 'alena.holligan@teamtreehouse.com' ],
However, it should be:
$contacts[] = ['name' => 'Alena Holligan', 'email'=> 'alena.holligan@teamtreehouse.com'];
Please notice the comma seperating 'name' and 'email', and the semicolon at the end of the expression.
Possible way to do this challenge (with better formatting):
$contacts = array();
$contacts[] = [
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
];
$contacts[] = [
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
];
$contacts[] = [
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
];
$contacts[] = [
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
];
BTW this code will still work even if you remove:
$contacts = array();
However, the PHP manual does not advise this, because if the array already contains some value (e.g. string from request variable) then this value will stay in the place and [] may actually stand for string access operator.

Antonio De Rose
20,885 Points<?php
//I think, you are mixing up with the square brackets with normal brackets.
//suggest, not to mix and, follow consistency.
//php 5.4 and above supports, square brackets
$contacts = array(
array('name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'),
array('name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'),
array('name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'),
array('name' => 'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com')
);
$contacts1 = [
['name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'],
['name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'],
['name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'],
['name' => 'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com']
];
echo "<pre>";
print_r($contacts);
echo "<pre>";
echo "<pre>";
print_r($contacts1);
echo "<pre>";

Dennis Boccardi
1,099 PointsThanks guys for the help I totally forgot about separating each element in the array with a comma thank you so much
Dennis Boccardi
1,099 PointsDennis Boccardi
1,099 PointsI figured it out. Nevermind.