Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial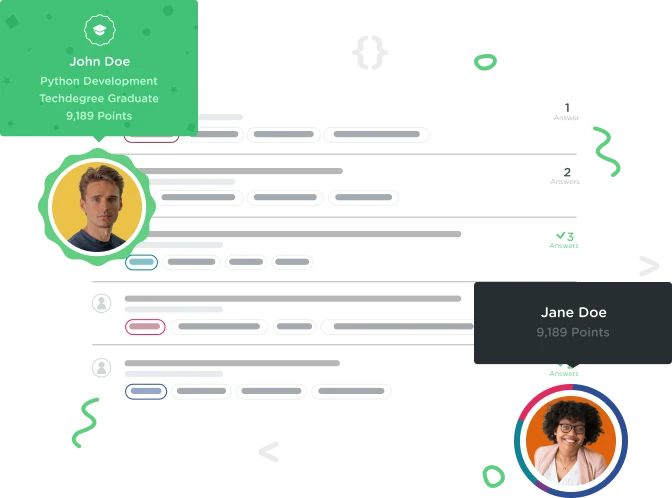

Diana Victoria Villada
9,543 PointsI have responded this challenge question and get the desired result but when i run the answer i get name is not defined
Create a method called eat. It should only take self as an argument. Inside of the method, set the is_hungry attribute to False, since the Panda will no longer be hungry when it eats. Also, return a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribute.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
print(f'{panda_one.name} eats {panda_one.food}')
self.is_hungry = False
panda_one = Panda('Bao Bao', 10)
panda_one.eat()
2 Answers
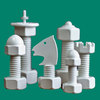
Steven Parker
230,970 PointsYou're close, here's a few hints:
- a class method should not directly reference an instance by name, use "self" instead
- make sure to
return
the string, you don't need toprint
anything - the string is missing the final punctuation
- for the challenge, you only need to define the class — you won't need to create an instance
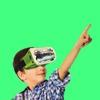
John Hill
Front End Web Development Techdegree Graduate 35,236 PointsHere's the code I got working. Had to remember to use self
on everything and to format the f-string correctly. I didn't use the 'Bao Bao' name anywhere, but it still accepted it as correct.
It's cool to see how using self
inside the eat()
method can access both the class attribute .food
and instance attribute .name
.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return f"{self.name} eats {self.food}."