Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial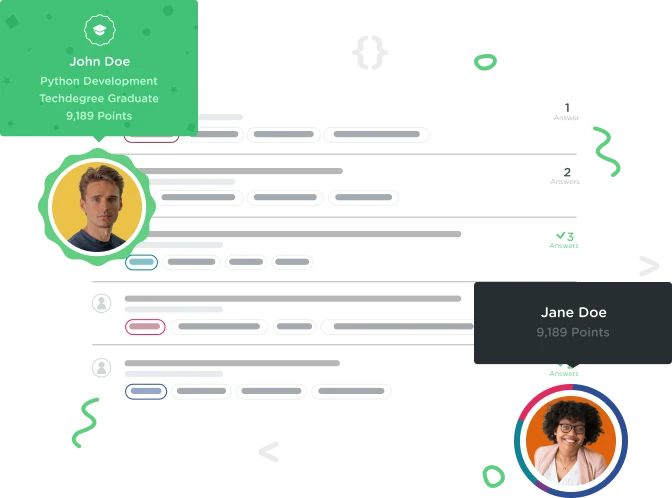
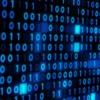
Alexander Davison
65,469 PointsI'd like to compare songs by their length (measured in whole seconds). Add the required methods for ==, <, >, <=, and >=
What's wrong with my code?
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __eq__(self, other):
return self.length == other.length
def __le__(self, other):
return self.length <= other.length
def __gr__(self, other):
return self.length > other.length
def __lt__(self, other):
return self.length < other.length
5 Answers

Christian Mangeng
15,970 PointsJust came upon this challenge, too, and thought I'd like to share my solution with you:
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __int__(self):
return int(self.length)
def __eq__(self, other):
return int(self) == other
def __gt__(self, other):
return int(self) > other
def __lt__(self, other):
return int(self) < other
def __ge__(self, other):
return int(self) >= other
def __le__(self, other):
return int(self) <= other
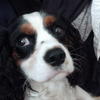
James J. McCombie
Python Web Development Techdegree Graduate 21,199 PointsI had a crack at this challenge, as Matt has already pointed out you had a method or two missing and your code did not implement comparing songs to ints, passed with:
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __int__(self):
return int(self.length)
def __eq__(self, other):
if isinstance(other, Song):
return self.length == other.length
elif isinstance(other, int):
return int(self) == other
else:
raise AttributeError
def __lt__(self, other):
if isinstance(other, Song):
return self.length < other.length
elif isinstance(other, int):
return int(self) < other
else:
raise AttributeError
def __le__(self, other):
if isinstance(other, Song):
return self.length < other.length or self.length == other.length
elif isinstance(other, int):
return int(self) <= other
else:
raise AttributeError
def __gt__(self, other):
if isinstance(other, Song):
return self.length > other.length
elif isinstance(other, int):
return int(self) > other
else:
raise AttributeError
def __ge__(self, other):
if isinstance(other, Song):
return self.length > other.length or self.length == other.length
elif isinstance(other, int):
return int(self) >= other
else:
raise AttributeError
Not entirely happy with the solution, but it passed. It may be an idea to add some type checking of sorts for the length property, may I also ask is this a new object orientated course you are following? as I really do not remember doing this the first time around
James
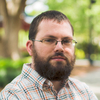
Kenneth Love
Treehouse Guest TeacherYou don't need that much code. What happens if you do, say, return int(self) > other
? does that reduce a lot of work?
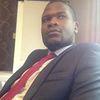
Tapiwanashe Taurayi
15,889 Pointsclass Song: def init(self, artist, title, length): self.artist = artist self.title = title self.length = length
def __int__(self):
return int(self.length)
def __eq__(self, other):
return int(self) == other
def __gt__(self, other):
return int(self) > other
def __lt__(self, other):
return int(self) < other
def __ge__(self, other):
return int(self) >= other
def __le__(self, other):
return int(self) <= other
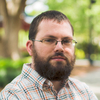
Kenneth Love
Treehouse Guest TeacherIt's not __gr__
, it's __ge__
.

Matt Juergens
16,295 PointsHaven't got there in python yet, but you are missing one of those methods. Hope I helped.