Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial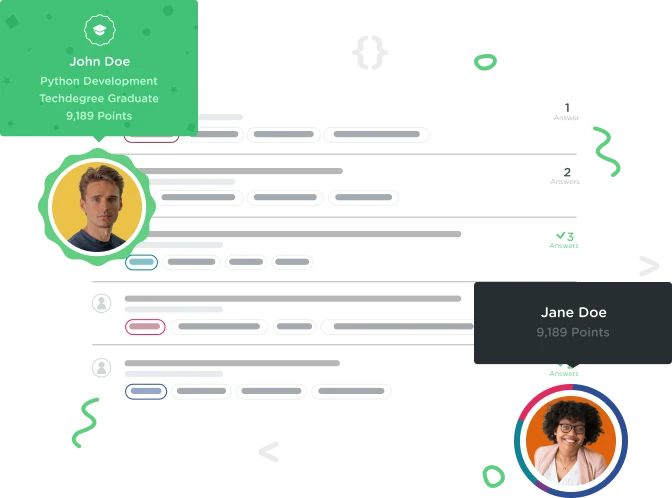

Jonas Troyer
10,912 PointsI'm getting a null exception at line 19. Not sure what to do here.
I looked up the answer to several other questions that are similar and I can't find what I'm doing wrong here. I'm not really sure how to solve this question. It's a small error somewhere, but I can't seem to find it.
Thanks
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String getTopic) {
this.topic = topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available ",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
// TODO: add getters for firstName and lastName
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost(User getAuthor, String getTitle, String getDescription) {
this.author = author;
this.title = title;
this.description = description;
}
// TODO: add a constructor that accepts the author, title and description
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("java");
String firstName = args[0];
String lastName = args[1];
User author = new User(firstName, lastName);
ForumPost post = new ForumPost(author, "Its a short post", "Thanks for being great and stuff");
forum.addPost(post);
}
}
1 Answer

andren
28,558 PointsThe issue is the names you give to parameters within the various constructors. Take the Forum
constructor for example:
public Forum(String getTopic) {
this.topic = topic;
}
Within the constructor you are meant to tell Java to set the topic
field variable equal to the parameter that got passed into the constructor. But you have named the parameter getTopic
, not topic
which is the name your code references.
If you changed the name like this:
public Forum(String topic) { // getTopic changed to topic
this.topic = topic;
}
Then that code would work.
The same issue is present in the constructor for the ForumPost
class as well and has to be fixed in the same way like this:
public ForumPost(User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}