Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial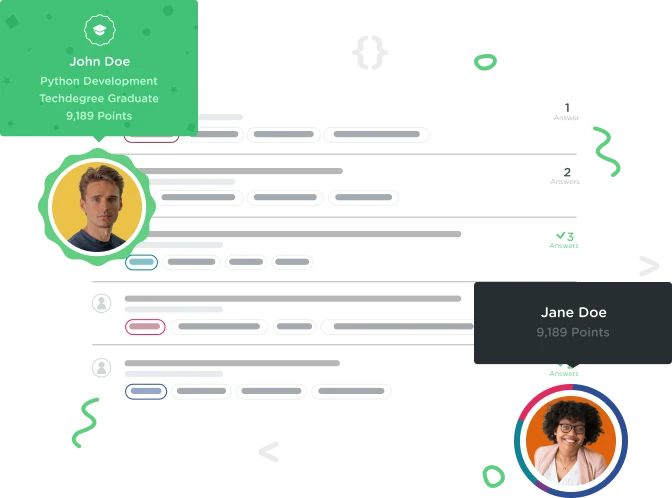
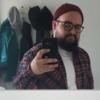
Gabe Olesen
1,606 PointsI'm stuck!
Hi All!
Can someone explain what I did wrong and what I'm not grasping? I want to fully understand this task before I move on. I'm on the right track but I'm missing something!
~Gabe
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if ('A' > 'M'){
return lastName.charAt(0);
}
if ('N' < 'Z') {
return lastName.charAt(1);
}
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers
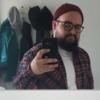
Gabe Olesen
1,606 PointsHi Ben,
You're correct, I didn't fully understand the question. I thought we had to check X char and return the index of the lasName but no, we just needed to check the first letter of the last name and the index starts at 0 so that would stay the same when checking both.
This was a good task, shame I didn't get it!
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lastName.charAt(0) <= 'M') {
lineNumber = 1;
} else if (lastName.charAt(0) >= 'M') {
lineNumber = 2;
}
return lineNumber;
}
Thanks again!
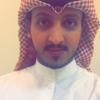
Fahad Mutair
10,359 PointsHi Gabe Olesen , In 2nd if statement you got logic error
else if (lastName.charAt(0) >= 'M')
because you have already checked for the M letter in the first statement
so you don't have to check it again ,
to solve this error just put greater than without equal (>)
else if (lastName.charAt(0) > 'M')

supniger
4,511 PointsThis Should do the trick:
public int getLineNumberFor(String lastName)
{
int lineNumber = 0;
char firstLineNumber = lastName.charAt(lineNumber);
if(firstLineNumber >= 'A' && firstLineNumber <= 'M')
{
return 1;
}
if(firstLineNumber >= 'N' && firstLineNumber <= 'Z')
{
return 2;
}
return lineNumber;
}
Ben Payne
1,464 PointsBen Payne
1,464 PointsI think you want to check if the first letter of the last name is greater than 'M' or less than 'M'. Then assign them to the proper line. Right now you are just checking that 'A' is greater than 'M' and 'N' < 'Z'.