Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial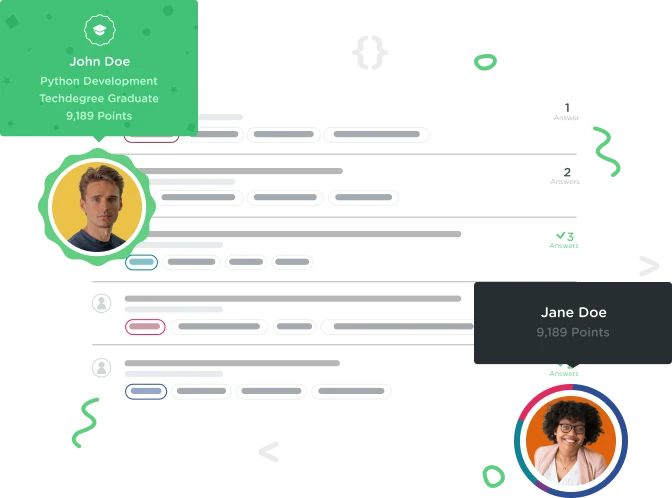

Esteban Salinas
1,873 PointsI'm stuck in the final task of this challenge
Here is my errors
./Main.java:15: error: constructor User in class User cannot be applied to given types; User author = new User(args[0]); ^ required: String,String found: String reason: actual and formal argument lists differ in length ./Main.java:18: error: constructor ForumPost in class ForumPost cannot be applied to given types; ForumPost post = new ForumPost(); ^ required: User,String,String found: no arguments reason: actual and formal argument lists differ in length Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 2 errors
I know it involves connecting User and ForumPost, but I don't know what it is
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public String getTopic() {
return topic;
}
public Forum(String topic) {
topic = "";
}
// Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
private String firstName;
private String lastName;
// TODO: add private fields for firstName and lastName
public User(String firstName, String lastName) {
firstName = "";
lastName = "";
// TODO: set and add the private fields
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
// TODO: add getters for firstName and lastName
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
private ForumPost(User author, String title, String description) {
this.author = author;
title = "";
description = "";
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
// Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost();
forum.addPost(post);
}
}
1 Answer

andren
28,558 Pointsconstructor User in class User cannot be applied to given types; User author = new User(args[0]); ^ required: String,String found: String reason: actual and formal argument lists differ in length
That error message is actually pretty descriptive. It is telling you that the User class only has a constructor that requires two string arguments to be passed in, and since you are only passing in one string argument that is invalid.
To get around this error message you have to actually supply two string arguments. The User class requires you to supply a firstName and a lastName as arguments. The firstName comes through the first argument to the program (so that part of your code is correct) but you also need the lastName which comes from the second argument.
So the User line is supposed to look like this:
User author = new User(args[0], args[1]);
The second error is essentially about the exact same thing. The ForumPost class requires you to pass in a User object and two strings as arguments, but you are not passing in anything.
The User object you should pass in is the one you make earlier in the code, and the two other string arguments are the tile and description of the ForumPost, which can be whatever you want.
Those are the only issues in your Main.java file but looking at your code I can see that you have other errors in your solution. Specifically the constructor for Forum, User and ForumPost are wrong.
You are meant to take the parameters passed in to the constructor and set the field variables in the class equal to them. And you do in fact do this correctly for the author
in the ForumPost:
private ForumPost(User author, String title, String description) {
this.author = author;
title = "";
description = "";
}
The assignment for the author
is correct, but your assignments for title
and description
is incorrect. They should all look pretty much identical to the author
assignment like this:
private ForumPost(User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
You have similar issues in all of the other constructors that you will have to fix before you can pass the challenge.