Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial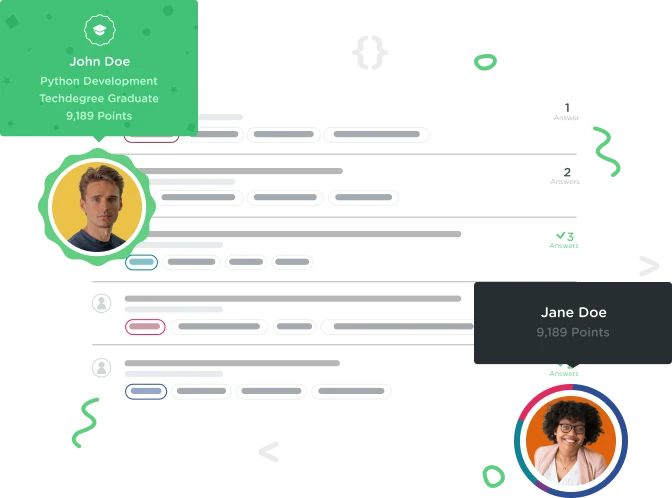
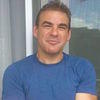
John Miller
4,007 PointsI'm stuck on these - can someone help
I'm lost, no idea where to go from here
// After you've completed the TODOs locally paste Main.java here
// After you've completed the TODOs locally paste Prompter.java here
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
9 Answers
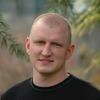
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo in this challenge it is assuming you are working locally in an IDE or even text editor of sorts. Though you can totally write it out in the different tabs for each file in the challenge itself it would be more ideal to do it in an IDE so you can see the runtime errors.
He is wanting you to turn the pseudocode into actual working code. You can rewatch the video titled "Teamwork". Craig walks through these files so you can see a lot of the code he wrote. Your code will look similar to what he has in there, with the exception of the differences he may have that is listed in the psuedocode file.
This challenge isnt just 1 simple answer and you are done. You will need to complete each step of the pseudocode then submit. Be sure if you work locally in an IDE, you name your files the same. The challenge will be looking for the same Class names.
And finally, once you get some beginning code together and start working on the logic of lets say the prompter class, if you get stuck come back here and post your code and we can work out the bugs together or even how you should go about the logic. You will learn MUCH MORE the more you are able to do yourself from start to finish.
You will want to start with a Main class with a public static void main(String[] args) method , that will be your codes start point.
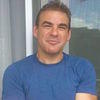
John Miller
4,007 PointsThank you, sometimes I'm a little confused by what they are asking for. So I need to actually write the code to implement a solution for the TODO's.
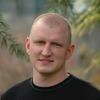
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsYes, you will be writing all the code necessary to make each TODO to pass listed in that pseudo-test file.
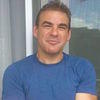
John Miller
4,007 PointsChris,
Am I to write the code from scratch or insert the code in the exiting Main, Template & Prompter files? I would assume I am to insert the code but his pseudo code has me a bit confused as it a bit vague on how and where it matches up to the TODO(s) in the code.
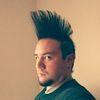
Ben Morse
6,068 Pointshey man,
how goes the challenge? where you able to complete it or are you still stuck?
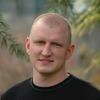
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsIn Craig's video he kind of casually gives you a brief overview what he did and what he needs done. Then "hands it off to you" to kind of simulate what happens between developers sometimes.
"Hey sorry I couldn't get all this work done before the meeting/flight/whatever, here is what I have. Gotta go!"
I think his vagueness was intentional as he wants you to try to come up with your own solution that outputs the same results. Doing it kind of throwing you "in the deep end" but it truly is an effective way to learn to code but can be frustrating.
If you are working in an IDE outside of the browser. I would say yes. Create and run the program on your own test what you can. Then copy paste the code into the each of the files provided ensuring your class names match up (otherwise you will get errors because class names generally match file names in java).
I believe when I went through this last bit, it did take a bit of time to work out some of the errors. But this is where testing your code in your own IDE comes in handy, especially for things like syntax errors. The logic errors can be harder to spot.
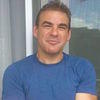
John Miller
4,007 PointsI get the following results when running my code in treehouse which I cannot duplicate in my IDE:
Enter Template: Enter Substitute for first prompt: Enter Substitute for second prompt: Censored Word: dork not allowed. Re-Enter Censored Word: dweeb not allowed. Re-Enter Your TreeStory:
Testing PASS_1 and then PASS_2
The relevant code in Prompter.java is:
public String promptForWord(String variable) throws IOException{
String userInput = null;
System.out.printf("Enter Substitute for %s: %n",variable);
userInput = mReader.readLine();
while (mCensoredWords.contains(userInput)) {
System.out.println("Censored Word: "+userInput+ " not allowed. Re-Enter");
userInput = mReader.readLine();
}
return userInput;
}
My Full Code is:
Main.java
public class Main {
public static void main(String[] args) {
Prompter promptR = new Prompter();
String story = null;
try {
story = promptR.newTemplate();
} catch (IOException e) {
e.printStackTrace();
}
Template tmpl = new Template(story);
promptR.run(tmpl);
}
}
Prompter.java
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String finalString = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", finalString);
}
public String newTemplate() throws IOException{
String newTmpl;
System.out.println("Enter Template: ");
newTmpl = mReader.readLine();
return newTmpl;
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String template : tmpl.getPlaceHolders()) {
String word = promptForWord(template);
words.add(word);
}
return words;
}
public String promptForWord(String variable) throws IOException{
String userInput = null;
System.out.printf("Enter Substitute for %s: %n",variable);
userInput = mReader.readLine();
while (mCensoredWords.contains(userInput)) {
System.out.println("Censored Word: "+userInput+ " not allowed. Re-Enter");
userInput = mReader.readLine();
}
return userInput;
}
}
I don't understand how these two words are slipping past my while block.
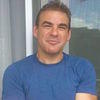
John Miller
4,007 PointsOkay, I figured out if you enter censored words multiple times you will overload the buffer and the invalid words will get by the while statement. I fixed this by importing the Scanner class and used this instead, now I am getting the following error: Bummer! java.util.NoSuchElementException (Look around Scanner.java line 862)
I guess the solution is to build a parser on the input from readLine
I'll do that next. I think this challenge should have had clearer instructions. Regardless of what "situation" the instructor is trying to demonstrate, he is not a work associate and available for clarification, perhaps he could be more clear and a little less clever.
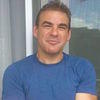
John Miller
4,007 PointsOk, I did a split on my user input and assigned the output to a String array then "tested" the first ('0') element. This worked and I am done - thanks everyone for your help.
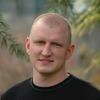
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsAwesome that you figured it out and went through the cycles of testing then troubleshooting your code repeatedly until you finally got your output working!
It is great when you get that last error working, "Yessssss!" feel accomplished the rest of the day.

Ashraf Belferd
Courses Plus Student 2,477 PointsHey , i am confused about why it gives null pointer exception with :
BufferedReader mReader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Write your story !!");
String story = null;
try {
story = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
used instead of :
Prompter promptR = new Prompter();
String story = null;
try {
story = promptR.newTemplate();
} catch (IOException e) {
e.printStackTrace();
}
in Main.java
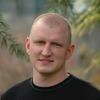
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsWhat is the newTemplate()
method *return*ing on the Prompter
class?
or better yet post the code of your Prompter class too.

Ashraf Belferd
Courses Plus Student 2,477 Pointshey Chris ,
that newTemplate()
is the same one that John Miller used in his Prompter.java class
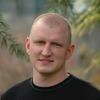
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsHey Ashraf Belferd,
I no longer have any code I wrote from when I did this series nor do I have the time right now to go through the videos to get each piece.
Though on Treehouse, if you start a java workspace. You can copy your code into their respective Class files then share that workspace and then I can see and run the code through the workspace.
Or
You can copy the Class code into blocks on here, though it may be better to start a new Post, if you are going to post all your classes. Because you could be having a completely different issue. :)