Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial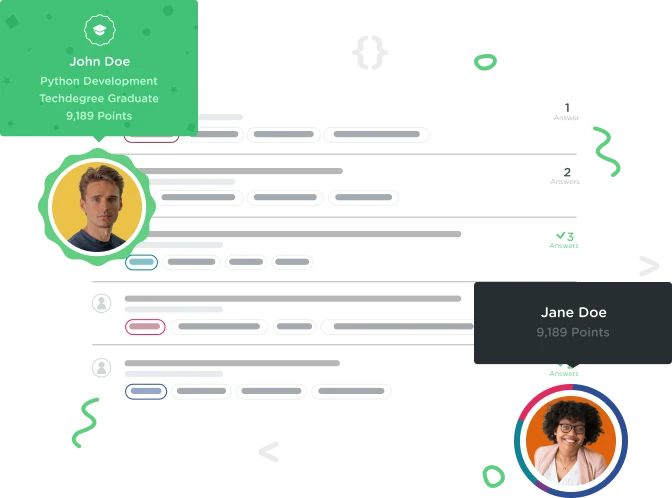
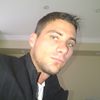
John Lukacs
26,806 PointsIn the code below, we have a simple array of contact names. We want to use the $contacts array to fill in the hardcoded
<?php
//edit this array
$contacts = array('Alena Holligan' => 'alena.holligan@teamtreehouse.com');
$another = array('Dave McFarland' => 'dave.mcfarland@teamtreehouse.com');
$another1 = array('Treasure Porth' => 'treasure.porth@teamtreehouse.com');
$another2 = array('Andrew Chalkley' => 'andrew.chalkley@teamtreehouse.com');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
I don't really know what this challenge is asking for
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
6 Answers

Phillip Legault
Courses Plus Student 18,888 Points$contacts = array(
array( "name" => "Alena Holligan", "email" => "alena.holligan@teamtreehouse.com", ),
array ( "name" => "Dave McFarland", "email" => "dave.mcfarland@teamtreehouse.com", ),
array( "name" => "Treasure Porth", "email" => "treasure.porth@teamtreehouse.com", ),
array( "name" => "Andrew Chalkley", "email" => "andrew.chalkley@teamtreehouse.com", )
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>" . $contacts[0]['name'] . " : " . $contacts[0]['email'] . "</li>\n";
echo "<li>" . $contacts[1]['name'] . " : " . $contacts[1]['email'] . "</li>\n";
echo "<li>" . $contacts[2]['name'] . " : " . $contacts[2]['email'] . "</li>\n";
echo "<li>" . $contacts[3]['name'] . " : " . $contacts[3]['email'] . "</li>\n";
echo "</ul>\n";
here is what I ended up with I see maybe the space for the quote?
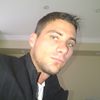
John Lukacs
26,806 Points<?php
//edit this array
$contacts = array('Name' => 'Alena Holligan');
$another1 = array( 'Name' => 'Dave McFarland');
$another2 = array('Name' => 'Treasure Porth');
$another3 = array('Name' => 'Andrew Chalkley');
Still does not work. What am I missing

Michael Stopa
14,965 PointsThis code worked ok for me:
$contacts = array(
array(
"name" => "Alena Holligan",
"email" => "alena.holligan@teamtreehouse.com",
),
array(
"name" => "Dave McFarland",
"email" => "dave.mcfarland@teamtreehouse.com",
),
array(
"name" => "Treasure Porth",
"email" => "treasure.porth@teamtreehouse.com",
),
array(
"name" => "Andrew Chalkley",
"email" => "andrew.chalkley@teamtreehouse.com",
)
);

Phillip Legault
Courses Plus Student 18,888 PointsI'm having the same issue. The first step is to make each person their own single item ASSOCIATIVE array, using 'name' as the key for these internal arrays. The above should be correct

Michael Stopa
14,965 PointsApologies for not having properly formatted the code;)

Phillip Legault
Courses Plus Student 18,888 Points$contacts = array(
$contact1 = array( "name" => "Alena Holligan", ),
$contact2 = array ( "name" => "Dave McFarland",),
$contact3 = array( "name" => "Treasure Porth", ),
$contact4 = array( "name" => "Andrew Chalkley",)
);
this worked for 1 of 3 I rechecked your example and it worked as well for 1 of 3 after removing the email

Jason Anello
Courses Plus Student 94,610 PointsHi Michael,
I formatted your code for you.
You can check this post for more info on posting code:

Michael Stopa
14,965 Points@Philip Legault Indeed, it looks like you cannot anticipate in this challenge;)
For task 3, I have the following code (nothing wrong with it in theory) but it's not passing:
echo "<ul>\n"; echo "<li>" . $contacts[0]['name'] . ":" . $contacts[0]['email'] . "</li>\n"; echo "<li>" . $contacts[1]['name'] . ":" . $contacts[1]['email'] . "</li>\n"; echo "<li>" . $contacts[2]['name'] . ":" . $contacts[2]['email'] . "</li>\n"; echo "<li>" . $contacts[3]['name'] . ":" . $contacts[3]['email'] . "</li>\n"; echo "</ul>\n";
Please advise.
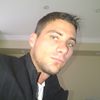
John Lukacs
26,806 PointsMan this challenge was hard you also have to omit all the <p> tags to get it to pass
Michael Stopa
14,965 PointsMichael Stopa
14,965 PointsThat was it! Great thanks, Philip.
A X
12,842 PointsA X
12,842 PointsAs of 2/17 this doesn't work for the challenge...error message is: "I do not see the required output. You should not be modifying the output at this point."
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsMaybe you have something different.
I just tried it and it works ok for me.