Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial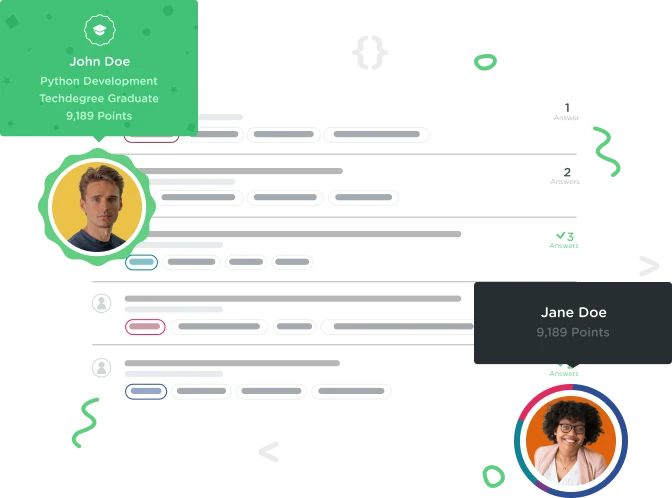

Unashe Mutambashora
3,433 Pointsincrement of number of laps
Any ideas on how I can increase the laps every time the method run_lap is called?
class RaceCar:
def __init__(self,color,fuel_remaining,laps = 0,**Kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for attribute,value in Kwargs.items():
setattr(self,attribute,value)
def run_lap(self.length):
laps = laps + 1
return fuel_remaining - (length * 0.0125)
3 Answers
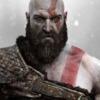
boi
14,242 PointsYou have a few problems with your code.
class RaceCar:
def __init__(self,color,fuel_remaining,laps = 0,**Kwargs): # 👈 An extra indent (error)
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for attribute,value in Kwargs.items():
setattr(self,attribute,value)
def run_lap(self.length): # 👈 Used a period (error)
laps = laps + 1 # 👈 Incorrect method to increment laps try (self.laps += 1)
return fuel_remaining - (length * 0.0125) # 👈 Incorrect method to use fuel_remaining. Also additional 0 in place
# Try (self.fuel_remaining -= length * 0.125)
Try to make these changes, and you are golden.
If problems still persists, tag me again by leaving a comment, and I will try to help out.

Unashe Mutambashora
3,433 PointsThanks. that was really helpful

Marion Lackie
1,753 PointsHi boi, this was really helpful. What I don't quite understand is why laps isn't set as an argument in the first line of the def_init_ method, along with the color and fuel_remaining arguments. I suspect this highlights my incomplete understanding of creating methods!!
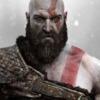
boi
14,242 PointsThe self.laps
argument should not be altered with, the self.laps
argument is a starting value and should be away from the user, suppose if we have set the self.laps
argument 👇
class RaceCar:
def __init__ (self, color, fuel_remaining, laps = 0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for attribute,value in kwargs.items():
setattr(self,attribute,value)
def run_laps(self,length):
self.laps+=1
self.fuel_remaining -= length * 0.125
Now the self.laps
argument can be set to anything, it would make no sense because the self.laps
attribute is like a condition, it will increase by default only if the run_laps
method is used.
Some attributes should be set behind the scenes, so it can be default or constant, that value should not be changed.
Note: In the above example even if the self.laps
argument is not given, it still can be changed by the user 👇
class RaceCar:
def __init__ (self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for attribute,value in kwargs.items():
setattr(self,attribute,value)
def run_laps(self,length):
self.laps+=1
self.fuel_remaining -= length * 0.125
>>> car = RaceCar("blue", 45, laps = 100)
>>> car.laps
100
This is because of the for_loop setattr
, to avoid this make these changes 👇
class RaceCar:
def __init__ (self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for attribute,value in kwargs.items():
setattr(self,attribute,value)
self.laps = 0 👈 #Set (self.laps) after the for loop
def run_laps(self,length):
self.laps+=1
self.fuel_remaining -= length * 0.125
>>> car = RaceCar("blue", 45, laps = 100)
>>> car.laps
0
Tag me if you need more help.

Marion Lackie
1,753 PointsNice!
That makes more sense to me now. Thanks for the detailed response :)
Unashe Mutambashora
3,433 PointsUnashe Mutambashora
3,433 PointsThanks for your response mate. I tried making those adjustments and still I am getting it wrong
class RaceCar: laps = 0
boi
14,242 Pointsboi
14,242 Points