Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial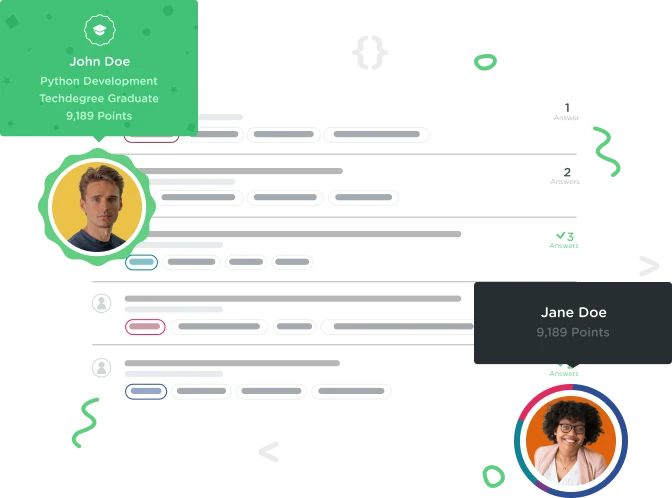

Youssef Moustahib
7,779 PointsIndexError!!
I am trying to get the "dinner" variable to give the user a random item from the shopping list but keep getting an index error. Where would it be best to place it? Can you fix my code to make it work? The indenting may be a bit wrong since I pasted it in here. Thanks. See code below:
import sys
import os
import random
shoplist = []
dinner = random.choice(shoplist)
def today(message, choice):
print("This machine will work out a random item for you to have at dinner today from your shoppinglist")
accept = input("Do you want to let it pick? Y/N").lower()
if accept == 'n':
sys.exit("no worries, enjoy making the decision yourself")
else:
print(message + "is ", "{}".format(choice))
sys.exit("enjoy")
def startagain():
try:
start = input("would you like to start again? Y/N").lower()
if not start.isalpha():
raise ValueError("Sorry only letters")
elif start != "y":
sys.exit("bye")
except ValueError as err:
print("Try again. {}".format(err))
else:
shoplist.clear()
main()
def helps():
print("Enter DONE to finish your list and see it \n"
"Enter SHOW to see your list \n"
)
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def addtolist(item):
clear()
if len(shoplist) < 1:
shoplist.append(item)
else:
try:
placement = int(input("where would you like to place your new item?\n"
"press enter to input it to the end of your list\n"
"?"))
except ValueError:
print("Error")
shoplist.append(item)
else:
shoplist.insert(placement - 1, item)
for index, item in enumerate(shoplist):
print("{}. {}".format(index + 1, item))
def show():
clear()
for index, item in enumerate(shoplist):
print("{}. {}".format(index + 1, item))
def main():
while len(shoplist) < 10:
try:
item = input("> ").lower()
if len(item) > 15 or len(item) <= 1:
raise ValueError("That item is too long to spell or is too short")
continue
elif not item.isalpha():
raise ValueError("Thats a number not an item..")
continue
elif item == "done":
sys.exit()
elif item == "show":
show()
continue
elif item == "help":
helps()
except ValueError as err:
print("Something has gone wrong. {}.".format(err))
else:
addtolist(item)
else:
print("you have reached the limit of 20 items")
today("Here it is:", dinner)
main()
1 Answer

Sebastian Rother
6,715 PointsFrom the Python Documentation:
random.choice(seq) - Return a random element from the non-empty sequence seq. If seq is empty, raises IndexError.
See also here for a more in-depth answer: https://docs.python.org/3/library/random.html
Just call the function later and only if you got two or more items (A random item of a list with only one item doesn't make much sense)
Youssef Moustahib
7,779 PointsYoussef Moustahib
7,779 PointsThank you!