Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial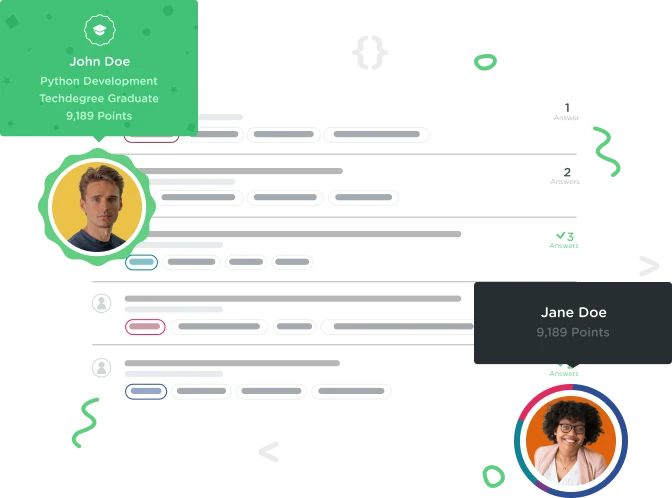

Barry Lam
14,406 PointsInfinite loop Happening with the Fly Instance
Not sure why this is happening. Every time I play the game, there is a infinite amount of flies being generated by the fly spawner. I checked the script, everything seems to be ok. But the game still does not stop even with when the while condition is met.
using UnityEngine; using System.Collections;
public class FlySpawner : MonoBehaviour {
[SerializeField]
private GameObject flyPrefab;
[SerializeField]
private int FlyMinimum = 12;
private float spawnArea = 25f;
public static int totalFlies;
// Use this for initialization
void Start () {
totalFlies = 0;
}
// Update is called once per frame
void Update () {
//While the total number of flies is less than the minimum...
while ( totalFlies < FlyMinimum ) {
//...then increment the total number of flies...
totalFlies++;
//...create a random position for a fly...
float positionX = Random.Range (-spawnArea, spawnArea);
float positionZ = Random.Range (-spawnArea, spawnArea);
Vector3 flyPosition = new Vector3(positionX, 2f, positionZ);
//..and create a new fly
Instantiate (flyPrefab, flyPosition, Quaternion.identity);
}
}
}
4 Answers
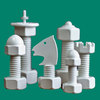
Steven Parker
241,970 PointsI'd be suspicious that some other part of the code was altering totalFlies or FlyMinimum. Have you tried logging both those values at the beginning of the loop?
Also, is there anywhere else in the code where flies can be spawned? Maybe it's not even happening in this function.

Barry Lam
14,406 PointsHey Steven,
Thanks for answer.
Found the error, turns out unity was pointing at an old instance of my fly spawner. Had to remove the game manager, remove the fly spawner and just rewrite.
Also curious to know why use a while loop when they could have used a if since we only want 12 flies active at one time?
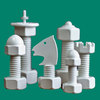
Steven Parker
241,970 PointsAn if would create at most one fly. So if you already had 2, you'd end up with 3.
The while continues to create flies until the count is equal to FlyMinimum (12). So if you already had 2, you still end up with 12.

Barry Lam
14,406 PointsAhh i see what you mean. Thanks for the clarification.