Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial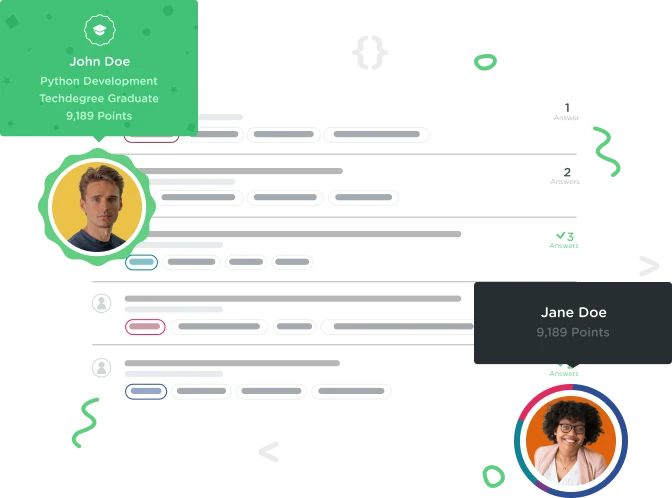

Jonathan Davidson
1,641 PointsIs there a way to get print to output multiple formated lines at once without having to use print for each line?
Is there a way to just use the print function once and keep the formatting without having to use print for each new line?
5 Answers

KRIS NIKOLAISEN
54,970 PointsPython has the new line character \n. For example
print("I like\ngreen eggs\nand ham")
will print
I like
green eggs
and ham

Vignesh Dhakshinamoorthy
1,788 PointsYou can use 3 double quotes:
multi_line = """I enjoy practice! I find it helps me to think better.
Without practice, my brain would probably not even work.
My code is getting more beautiful every single day!
"""
print (multi_line);
Sometimes, you had want variables to be printed as part of your multi-line string. This is possible with a little extra work using the .format() method.
verb = 'think';
noun = 'brain';
adj = 'beautiful';
multi_line = """I enjoy practice! I find it helps me to {VERB} better.
Without practice, my {NOUN} would probably not even work.
My code is getting more {ADJECTIVE} every single day!
""".format(VERB=verb, NOUN=noun,ADJECTIVE=adj);
print (multi_line);

Lucas Taylor
125 PointsWould this print breaks where you have the break on the lines in the code?

Enrica Fedeli-Jaques
6,773 PointsHi, I wrote print("I enjoy practice! I find it helps me to", verb, "better. Without practice, my", noun, "would probably not even work. My code is getting more", adj, "every single day!") which printed I enjoy practice! I find it helps me to run better. Without practice, my nun would probably not even work. My c ode is getting more black every single day!

Enrica Fedeli-Jaques
6,773 Pointsoh sorry, I think I missed that you still wanted the text on multiple lines, and my code was in one line, my previous comment might be not relevant

Carolina Romero Gutierrez
Python Development Techdegree Student 277 PointsHi Enrica, your comment helped me a lot, because I wrote a one sentence string but I think I made some commas mistakes or I don't know what happened but now that I looking at your work I did it right this time. Thanks for your help.

Arnold Ganga
1,698 Pointsyes there is a way that used in my practice solution below
verb = input("Tell us one verb about what area does practice help you: ") noun = input("tell us one noun about what would be affected if you did not practice: ") adjective =input("Okay tell us one adjective about what your code is doing because of practice: " )
print(" I enjoy practice! I find it helps me to {} better.\n Without practice, my {} would not even work.\n My code is getting more {} every single day !".format(verb, noun,adjective))
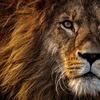
nathaniel marquez
223 Pointsyou could put the whole response in one print function as follows.
verb = input("Give me a verb.")
noun = input("Give me a noun.")
adjective = input("Give me an adjective.")
print("I enjoy practice! I find it helps me to, verb, better.", "\n", "Without practice, my", noun, "would not even work.", "\n", "My code is getting more", adjective, "every single day!")