Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial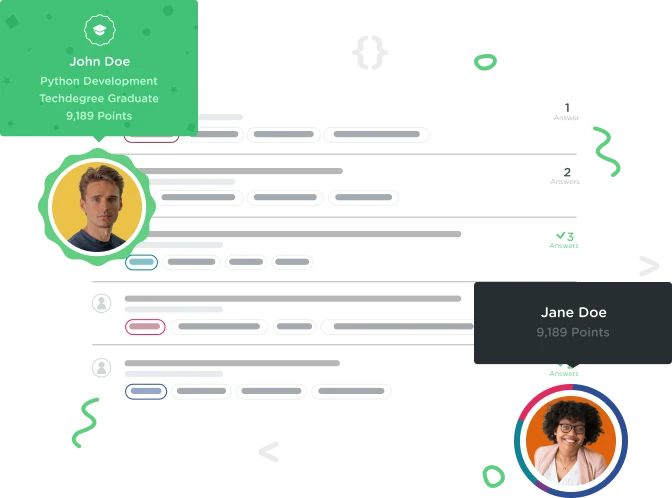
4 Answers
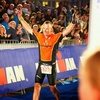
Steve Hunter
57,712 PointsHi there,
There's 4 parts to this question. First is Declare a variable that is named the camel-cased version of "first name". Store the user's first name into this new variable using console.readLine. For that you need to create a String called firstName
and ask for user input, as it says. That line looks like:
String firstName = console.readLine("Enter your first name: ");
Next, the question is Declare another variable, naming this one the camel-cased version of "last name". Use console.readLine to store the user's last name into this new variable. THat's pretty much the same as above:
String firstName = console.readLine("Enter your first name: ");
String lastName = console.readLine("Enter your last name: ");
Next you want to output the first name Using the console's printf method, display a message that says, "First name: ", followed by the first name that the user has entered. That's using string interpolation to dd the value held in firstName
where the %s is in the output string. Like this:
String firstName = console.readLine("Enter your first name: ");
String lastName = console.readLine("Enter your last name: ");
console.printf("First name: %s", firstName);
And the last tak is similar to the third Using the console's printf method, display a message that says, "Last name: " followed by the last name that the user has entered.
String firstName = console.readLine("Enter your first name: ");
String lastName = console.readLine("Enter your last name: ");
console.printf("First name: %s", firstName);
console.printf("Last name: %s", lastName);
I hope that helps you.
Steve.
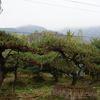
Learning coding
Front End Web Development Techdegree Student 9,937 PointsOk, I am trying to understand the vocabulary here;
console.printf = method? (or function) First name = variable? firstName = parameter?
console.printf("First name: %s", firstName); console.printf("Last name: %s", lastName);
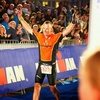
Steve Hunter
57,712 PointsLet's try to break this down.
In the first line console
is an instance (object) of the Console
class. Then, printf
is a method (or function) of the Console
class instances. A method and function are interchangeable names for the same thing.
Next we are creating a variable of type String
called firstName
. It could be called an instance of the String
class holding the value you assign to it, but let's not. The variable firstName
holds a string like "Steve
".
In the last line, you are using the string formatting capability of printf
. This allows you to insert the value of a variable into a string that you define. You are passing two things into the printf
method/function. First is the string you want to have a value inserted into, "First name: %s
", then the variable whose value you want to insert at the %s
position, firstName
. You can call both those things parameters when used in this way. A value passed into a method is a parameter. So, on one line, firstName
is a string variable, next you are using the same string variable as a parameter of the printf
method.
Let me know if that doesn't make sense and I'll have a better try!
Steve.
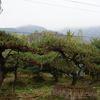
Learning coding
Front End Web Development Techdegree Student 9,937 PointsHi Steve,
After reading your answer a few time's I still don't understand it fully, but it helped me understand more. That's good for now. Thanks for your extensive answer.
RenΓ©
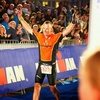
Steve Hunter
57,712 PointsIf you let me know what's still not clear, I will try again!
Steve.
tapiwa chauruka
2,502 Pointstapiwa chauruka
2,502 Pointsthanks a lot steve. it worked perfectly
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points