Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial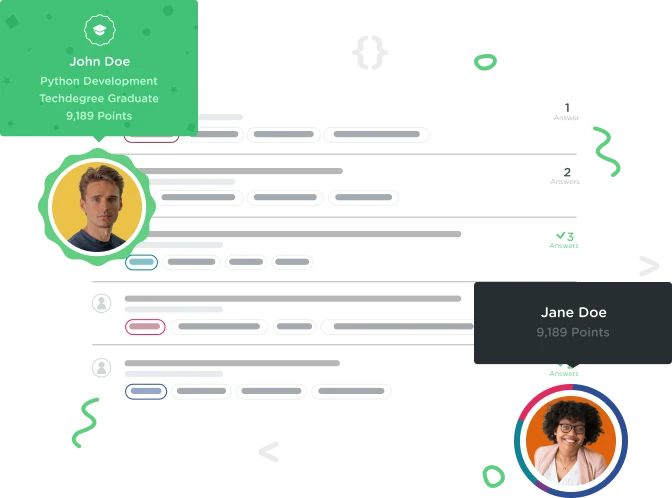

Zijun Tong
1,291 PointsIt looks like task 1 is not passing
Task 1 is to return discountCode in upper case. Task 2 is to throw illegal argument exception if it's not a letter or $. The following code says task 1 is not passing anymore, and I don't know why.
private String normalizeDiscountCode(String discountCode2){ this.discountCode = discountCode2.toUpperCase(); for (char letter : discountCode2.toCharArray()){ if (! Character.isLetter(letter) || letter != '$'){ throw new IllegalArgumentException("Invalid discount code"); } }
return this.discountCode;
}
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode(String discountCode2){
this.discountCode = discountCode2.toUpperCase();
for (char letter : discountCode2.toCharArray()){
if (! Character.isLetter(letter) || letter != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
return this.discountCode;
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode;
normalizeDiscountCode(this.discountCode);
}
}
2 Answers
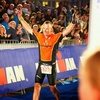
Steve Hunter
57,712 PointsHi there,
There's a few issues in here.
First, don't set this.discountCode
in this method. That gets done in the applyDiscountCode()
method already.
Next, you've set the member variable, i.e. this.discountCode
, before doing the validation so incorrect codes are capable of being put into the member variable, rather than the IllegalArgumentException
catching these invalid codes.
In applyDiscountCode()
you have called normalizeDiscountCode
but not assigned it's returned value back into this.discountCode
. You have also passed the member variable into the second method, rather than the parameter. The applyDiscountCode()
method receives the code for processing. It sends it to normalizeDiscountCode()
for validation, then what's returned into the member variable. Your method hasn't used the parameter at all. So, you need to send the parameter to normalize...
and assign the returned value into this.discountCode
. That would look like:
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
Back to normalizeDiscountCode()
; first sort the returned value. That is the parameter the method receives (sent by applyDiscountCode
) made uppercase. So, we can handle the receipt and onward transmission of the code with this:
private String normalizeDiscountCode(String discountCode){
return discountCode.toUpperCase();
}
Now we need to figure out the validation. You've pretty much got that spot on already! However, you used OR, when I think this needs an AND. Tidying up, taking all the above into account, and you get something like this:
private String normalizeDiscountCode(String discountCode){
for (char letter : discountCode.toCharArray()){
if (! Character.isLetter(letter) && letter != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
I hope that helps. Give me a shout if you need more on this - there's a lot in there! I hope it all works, too!
Steve.

Zijun Tong
1,291 PointsHi Steve, that worked perfectly!! Thanks for the detailed explanation =)
Zijun
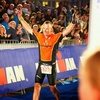
Steve Hunter
57,712 PointsNo problem!