Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial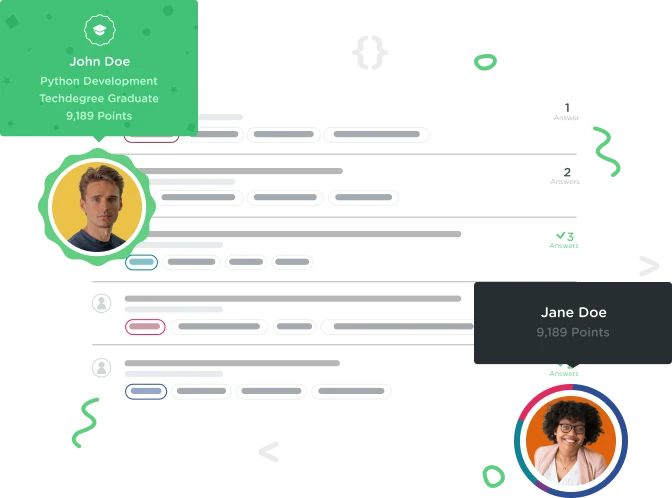

zfusdjitsz
2,797 PointsI've tested this in a python shell and it works. Can anyone offer some insight?
def time_machine(integer,time_string):
if time_string == "minutes":
return starter + datetime.timedelta(minutes=integer)
elif time_string == "seconds":
return starter + datetime.timedelta(seconds=integer)
elif time_string == "hours":
return starter + datetime.timedelta(hours=integer)
elif time_string == "days":
return starter + datetime.timedelta(days=integer)
else:
return starter.replace(year = starter.year + integer)
Also, I'm pretty sure there's a way to use the value of a variable instead of having to do all those ifs but I think I'm having a brain fart. Essentially I would've liked to do this:
if time_string == "years":
return starter.replace(year = starter.year + integer)
else:
return starter - datetime.timedelta(time_string=integer)
Just couldn't figure out how to dereference. Maybe dereference isn't the term but you know what i mean :)
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Kurt,
For your second code sample you could create a dictionary with the 2 arguments and then unpack that dictionary into keyword arguments.
This {time_unit : integer}
will produce something like {'hours': 5}
Then when you unpack it like this datetime.timedelta(**{time_unit : integer})
It would be as if you did this datetime.timedelta(hours=5)
I'm not sure if this is a good way to do it but it seems to work.
I wasn't sure either if there was a nice way to handle the "years" case. My idea was to simply change the time unit to days and convert the integer by multiplying by 365.
def time_machine(integer, time_unit):
if time_unit == "years":
time_unit = "days"
integer *= 365
return starter + datetime.timedelta(**{time_unit : integer})
I was able to get your first code sample to pass by changing the final return to this:
return starter + datetime.timedelta(days=integer*365)
Unfortunately, I'm not sure why starter.replace()
didn't work out.
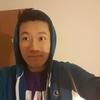
Anthony Liu
11,374 PointsFor the 'years' string, you can convert the number of years into number of days so you are able to use .timedelta(days = new integer)
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsLooking at this further, there is a difference between the two. I realized I'm not taking into account leap years yet it passes the challenge.
Kurt, your idea of year replacement seems like it would be the easiest and most accurate yet it doesn't pass the challenge.
If I run both solutions with an input of 10 years I get the following results: