Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial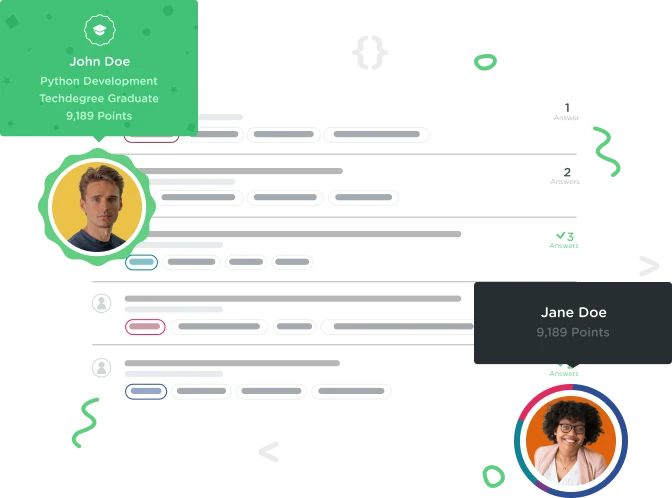
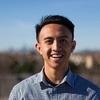
Gendarme Docena
1,509 PointsJava Objects, Conference Registration Assistant
Hi, I'm unsure on how to rewrite my finished code. Can someone please take a look at this?
The directions tell me to: "Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z."
I thought I did it correctly based on an if/else statement. I received 0 errors but a simple revision that says: "Make sure you use the lastName that was passed in to the method. You can get characters using the charAt method on Strings"
I thought I would use "lineNumber" because that's the integer being used instead of "lastName". Also, I'm not sure what the statement means "..using the charAt method on Strings." I didn't see that used in the last video, so I guess that's my problem.
public class ConferenceRegistrationAssistant {
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lineNumber >= 'A' && lineNumber <= 'M') {
System.out.println("1");
} else {
System.out.println("2");
} return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
4 Answers

andren
28,558 PointscharAt is both used and explained in the last video, to be more specific Craig first starts talking about it at around 2:10 in the Prompting for Guesses video. Maybe you skipped over the video by accident?
Anyway to summarize the relevant portion of the video the charAt method is simply a method you can use on strings to pull out a single char from the string at a given index. So by using charAt(0) for example you get access to the first character in a string.
To solve this task you have to compare the first character of the lastName string to 'A' and 'M'. The lineNumber variable is there because the method is meant to return the line number, you are supposed to set that variable to 1 if the first character of the last name is between 'A' and 'M' and set it to 2 if it isn't. And then return the lineNumber variable at the end.
So the two main issues with your current solution is that you are not actually comparing the lastName variable like you are supposed to, and the fact that you are printing 1, and 2. Instead of setting the lineNumber variable equal to 1 or 2, which is what the challenge is actually asking you to do.
If you fix those two issues you will be able to complete the challenge. If you still have trouble solving it after reading the hints I have given then I can post the solution for you, but ideally you should try to solve it yourself first.

Bhushan Sabadra
Courses Plus Student 1,646 Pointscool here is your answer
if (lastName.charAt(0) >= 'A' && lastName.charAt(0) <= 'M') { System.out.println("1"); } else { System.out.println("2"); }
"charAt(zero) not O"
else you have all the logic up to mark just this change you are done..... enjoy coding

Rohit Narula
1,273 Pointspublic int getLineNumberFor(String lastName) { int lineNumber = 0; /* lineNumber should be set based on the first character of the person's last name Line 1 - A thru M Line 2 - N thru Z
*/
String one = "ABCDEFGHIJKLM";
String two = "NOPQRSTUVWXYZ";
boolean checkLetter = one.indexOf(lastName.charAt(0)) != -1;
if (checkLetter){
return lineNumber = 1;
} else {
return lineNumber = 2;
}
}

Paul Vickers
3,380 PointsThanks for you help here, it helped me figure it out for myself and understand it better :)
Gendarme Docena
1,509 PointsGendarme Docena
1,509 PointsOkay sorry, yeah I'm not sure what happened but I did see charAt used in the last video.
I've been stuck on this for the past hour trying to understand this. So to fix this, do I write the code similarly to the "Prompting for Guesses" video? Like how Craig imported a scanner and made a separate boolean?
Honestly I'm still pretty confused. The video doesn't seem to be similar to the exercise because it has the extra java.game tab. I don't work on anything in the "Example.java" tab right? It says it's only for demonstration use.
andren
28,558 Pointsandren
28,558 PointsYou don't need to use a scanner because the lastName is passed in as a parameter to the method, so that variable already exists, and you do indeed not need to touch the example.java file at all.
Honestly you don't actually have to change the code you have already written all that much, just change what you are actually comparing in the if statement to be the first char of the lastName variable instead of the lineNumber variable, and change the code in the if statement from printing 1 or 2 to code that sets the lineNumber variable to 1 or 2. And that's pretty much all you have to do to solve this challenge.
If you are still unable to solve the challenge with those hints then I have posted the solution for you here so that you can see what the answer is. But try to solve it yourself before looking at it.
Gendarme Docena
1,509 PointsGendarme Docena
1,509 PointsOh wow, I just got it. Thanks for the hints!
It took awhile. Man I am slow at this! :(
andren
28,558 Pointsandren
28,558 PointsIt's perfectly normal to be a bit "slow" with these things in the beginning, practice makes perfect as they say. It certainly took me a while before I really started to wrap my head around how to program in Java, and it required quite a bit of coding practice before it "clicked" with me, but once it did it became a lot easier to use and learn.
So keep at it, I wish you good luck ahead.