Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial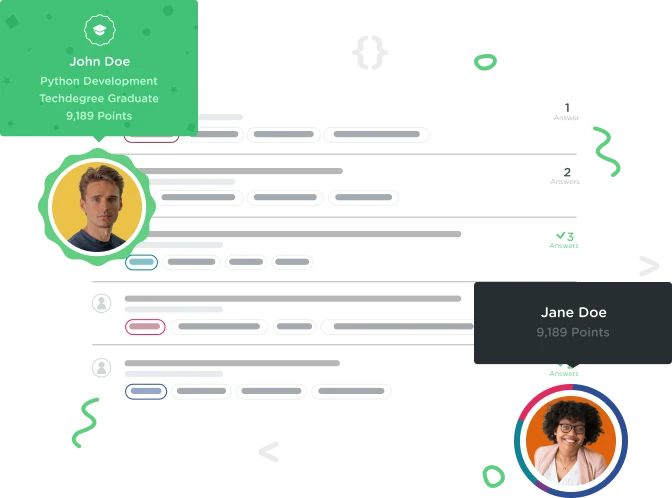

James Mackey
Courses Plus Student 994 PointsLine 16: Cannot figure out what wrong around the word quit?
using System;
namespace Treehouse.FitnessFrog { class Program { static void Main() {
int runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type "quit" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
}
else
{
int minutes = int.Parse(entry);
if(minutes <=10)
{
Console.WriteLine("Better than nothing, right?");
}
else if (minutes <=30)
{
Console.WriteLine("Way to go hot stuff!");
}
else if (minutes <=60)
{
Console.WriteLine("You must be a ninja warrior in training!");
}
else (minutes >=60)
{
Console.WriteLine("Ok now you are just showing off!!");
}
}
runningTotal = runningTotal + minutes;
// Add minutes exercised to total
// Display total minutes exercised to the screen
Console.WriteLine("You've entered " + runningTotal + " minutes");
}
// Repeat until the user quits
}
Console.WriteLine("GoodBye!");
}
}
1 Answer
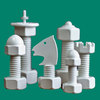
Steven Parker
231,236 PointsThe word quit is outside the string and confuses the compiler.
Your string is defined by quotes ("), so if you also put quotes around a word in the string, it is considered not part of the string. To make the quotes (and the word inside them) part of the string you must escape the quotes with backslash (). Here's an example:
Console.Write("Enter how many minutes you exercised or type "quit" to exit: "); // original
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: "); // escaped
Notice how the syntax coloring clearly shows that quit in the first line is not part of the string.
Now that issue is taken care of, I noticed two other things that might need fixing:
- the line with "
else (minutes >=60)
" seems to be missing an "if
", but it could also be left as just "else
" since the other cases are already accounted for. - the line with "
Console.WriteLine("GoodBye!");
" is actually outside the method, and should be moved inside.
James Mackey
Courses Plus Student 994 PointsJames Mackey
Courses Plus Student 994 PointsProgram.cs(42,16): error CS1525: Unexpected symbol
{' Program.cs(42,17): warning CS0642: Possible mistaken empty statement Program.cs(57,246): error CS1525: Unexpected symbol
end-of-file'Compilation failed: 2 error(s), 1 warnings
How is there an unexpected "{" here?? Do I not need this?
and i have no idea what line 57 means? Is it not he end of file there with a "}"