Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial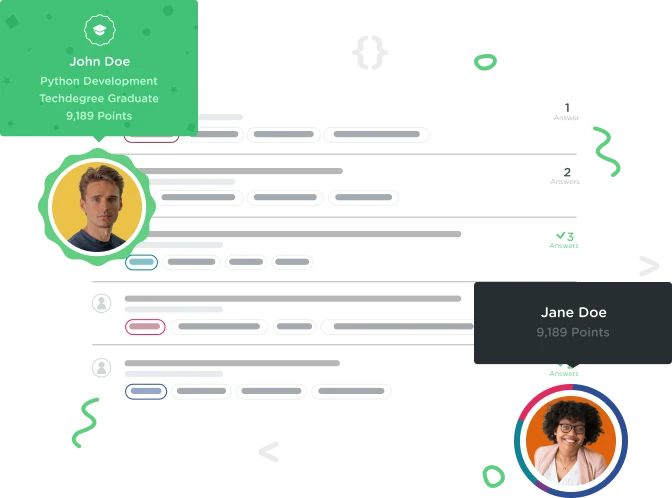
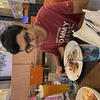
Arnav Goyal
1,167 PointsList comprehension problem
The purpose is to print out all possible coordinates of a given cuboid with specified dimensions(x,y,z) to form a multidimensional list. However the sum of coordinates should not be equal to a given number 'n'. Note: Can be done using for loops but trying to learn list comprehension better:) x,y,z,n are standard inputs Can you help with the error in this code
coordinates = print([[x,y,z] for x in range(x+1) for y in range(y+1) for z in range(z+1)if x + y + z != n])
1 Answer
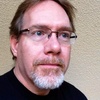
Chris Freeman
Treehouse Moderator 68,426 PointsHey Arnav Goyal, neat challenge! There are two errors in your code.
-
print
returnsNone
after output a value, socoordinates
would always beNone
- the comprehension does not seem to like implied nested loops where the loop variable i is the same name as range variable. [I need to dig a bit more to find out why]
# why does this work:
>>> x = y = 3
>>> x , y
(3, 3)
>>> [[x] for x in range(x+1)]
[[0], [1], [2], [3]]
# but this does not work:
>>> [[x,y] for x in range(x+1) for y in range(y+1)]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 1, in <listcomp>
UnboundLocalError: local variable 'y' referenced before assignment
To solve your problem, change the loop variables:
x = y = z = 3
n = 6
coordinates = [[x0 ,y0 ,z0]
for x0 in range(x+1)
for y0 in range(y+1)
for z0 in range(z+1)
if x0 + y0 + z0 != n]
print(coordinates)
# outputs
# [[0, 0, 0], [0, 0, 1], [0, 0, 2], [0, 0, 3], [0, 1, 0], [0, 1, 1], [0, 1, 2], [0, 1, 3], [0, 2, 0], [0, 2, 1], [0, 2, 2], [0, 2, 3], [0, 3, 0], [0, 3, 1], [0, 3, 2], [1, 0, 0], [1, 0, 1], [1, 0, 2], [1, 0, 3], [1, 1, 0], [1, 1, 1], [1, 1, 2], [1, 1, 3], [1, 2, 0], [1, 2, 1], [1, 2, 2], [1, 3, 0], [1, 3, 1], [1, 3, 3], [2, 0, 0], [2, 0, 1], [2, 0, 2], [2, 0, 3], [2, 1, 0], [2, 1, 1], [2, 1, 2], [2, 2, 0], [2, 2, 1], [2, 2, 3], [2, 3, 0], [2, 3, 2], [2, 3, 3], [3, 0, 0], [3, 0, 1], [3, 0, 2], [3, 1, 0], [3, 1, 1], [3, 1, 3], [3, 2, 0], [3, 2, 2], [3, 2, 3], [3, 3, 1], [3, 3, 2], [3, 3, 3]]
Post back if you need more help. Good luck!!!