Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial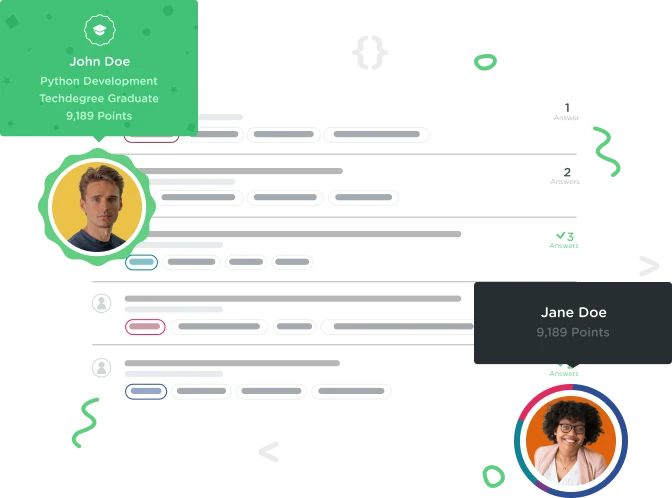
John McErlain
12,508 PointsList not accepting *args
The following code returns the correct answer for a variety of different lists and tuples (for float types) on my computer. However, in the code challenge, there is an error message stating the list only takes one argument and I do not understand 'why'.
Thank-you
# If you need help, look up datetime.datetime.fromtimestamp()
# Also, remember that you *will not* know how many timestamps
# are coming in.
import datetime
def timestamp_oldest(*args):
timestamps = list(*args)
timestamps.sort()
conversion = datetime.datetime.fromtimestamp(timestamps[len(timestamps)-1], tz = None)
return conversion
3 Answers
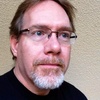
Chris Freeman
Treehouse Moderator 68,426 PointsYour very close. When using *args
as a parameter, you then reference it without the "*":
import datetime
def timestamp_oldest(*args):
timestamps = list(args) #<-- removed "*"
timestamps.sort()
#conversion = datetime.datetime.fromtimestamp(timestamps[len(timestamps)-1], tz = None)
conversion = datetime.datetime.fromtimestamp(timestamps[0]) #<-- "oldest" is lowest number
return conversion
John McErlain
12,508 PointsI appreciate your response Chris.
I have a follow-up question to this regarding the type of arguments passed into the function. When I test the function with: args = 999999,34,543267843,344565,894,9000873,32505000000,9872, I get a type error that "an integer is required". Does this have something to do with unpacking a tuple?
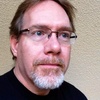
Chris Freeman
Treehouse Moderator 68,426 PointsWhen calling a function with a arguments in a tuple, you also have to unpack the tuple on the call. The *-expand can be used on the call as well as in the function definition.
# define arguments as tuple
In [78]: args = 999999,34,543267843,344565,894,9000873,32505000000,9872
# try calling function with tuple (single argument that is a tuple)
In [79]: timestamp_oldest(args)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-79-1986d3f6ae1b> in <module>()
----> 1 timestamp_oldest(args)
<ipython-input-77-4b4cf9b1e3a2> in timestamp_oldest(*args)
3 timestamps.sort()
4 #conversion = datetime.datetime.fromtimestamp(timestamps[len(timestamps)-1], tz = None)
----> 5 conversion = datetime.datetime.fromtimestamp(timestamps[0]) #<-- "oldest" is lowest number
6 return conversion
7
TypeError: an integer is required (got type tuple)
# call function with * expanded tuple
In [82]: timestamp_oldest(*args)
Out[82]: datetime.datetime(1969, 12, 31, 16, 0, 34)
# call function with expanded arguments
In [83]: timestamp_oldest(999999,34,543267843,344565,894,9000873,32505000000,9872)
Out[83]: datetime.datetime(1969, 12, 31, 16, 0, 34)
John McErlain
12,508 PointsThis was very helpful. Thank-you Chris!