Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial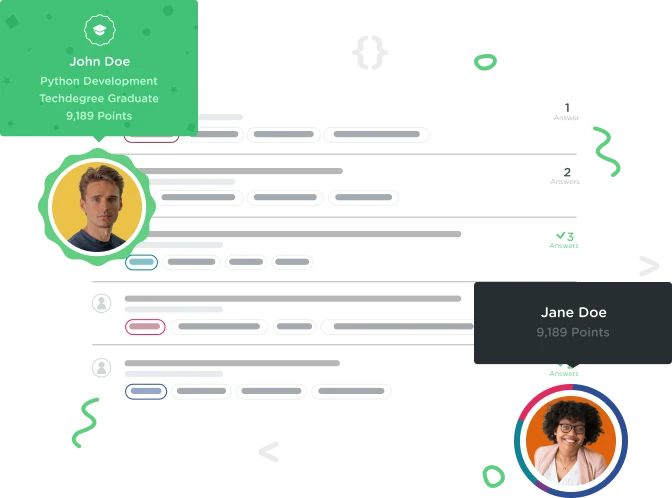
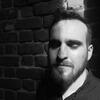
matthew mahoney
Python Development Techdegree Student 2,536 PointsMaster_Ticket.py
So I messed up this code somewhere along when I added the "else" statement after the "try" statement... i know it has something to do with the indentation but I'm not sure.
TICKET_PRICE = 10
tickets_remaining = 100
user_name = input("Hello there, what should we call you? ")
while tickets_remaining >= 1: print(f"Okay, {user_name}, tickets are {TICKET_PRICE} each. There are {tickets_remaining} tickets available.") number_of_tickets = input("How many would you like? ") try: number_of_tickets = int(number_of_tickets) except ValueError: print("Oh no! Doesnt look like you like to play by the rules! Try again! ") else: price = int(number_of_tickets) * TICKET_PRICE print(f"The total due for that many tickets would be ${price}.") buy = input(f"Would you like to continue? Your card will be charged ${price}. y/n? ") if buy == "y": print("SOLD!") tickets_remaining -= int(number_of_tickets) print(f"There are now {tickets_remaining} tickets remaining for purchase, just in case you change your mind.") else: print(f"Thanks {user_name}, for wasting my prescious bits, for nothing!") print(f"Sorry {user_name}, we're all sold out of tickets :(.")
1 Answer
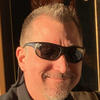
Peter Vann
36,427 PointsHi Matthew!
This is probably overkill, but at least it should give you a better handle on proper spacing in the try/except/else blocks...
(And make sure your indents are precisely 4/8/12/16/20/etc... spaces only - don't use tabs.)
Here is a version of my solution (which goes beyond the challenge) with error handling:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
valid = False
while valid != True:
client_name = input("What is your name? ")
if (len(client_name) == 0):
print("*** INVALID SELECTION ***")
else:
try:
val = int(client_name) ### IF THIS FAILS, client_name is a string - the desired value type ###
except ValueError: ### BACKWORDS LOGIC HERE ###
valid = True
else:
print("*** INVALID SELECTION ***")
valid = False
while valid != True:
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
try:
val = int(num_ticket)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val <= tickets_remaining:
valid = True
else:
print("*** ERROR ***: There {} only {} ticket{} remaining. Try again.".format(verb, tickets_remaining, remaining_s))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
If you notice, in one section I sort of use ValueError backwards, but it makes it work. - I try to cast clientName to an int, and when it errors, I know it's a string (and therefore valid when it errors!?!) Again, kind of backwards logic, but sometimes you have to think outside-the-box!?! LOL
From this thread:
https://teamtreehouse.com/community/can-someone-help-me-with-this-python-script
You can test it here:
https://www.katacoda.com/courses/python/playground
I hope that helps.
Stay safe and happy coding!
matthew mahoney
Python Development Techdegree Student 2,536 Pointsmatthew mahoney
Python Development Techdegree Student 2,536 PointsWonderful as always, Peter. So the problem was my indenting for the else block? I suspected so.
Thanks, Matt