Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial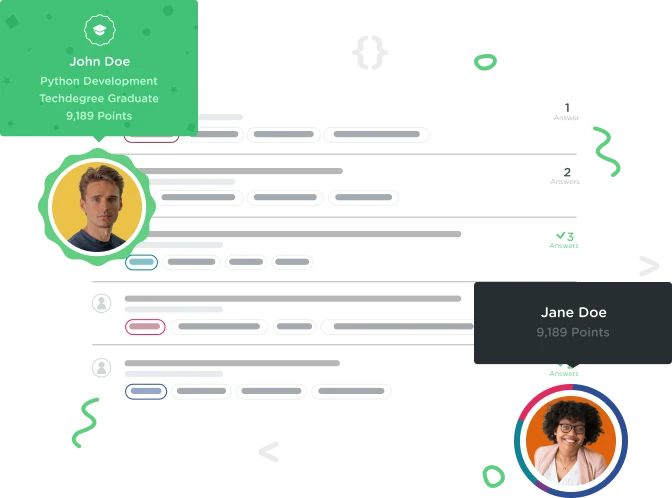

Péter Simon
1,054 PointsMorse code challenge - my solution works in a Workspace, but not accepted
I tested out my solution, works in a workspace, but does not pass as a solution. Essentially I convert each element of the array then add it to a string, then at the end return the string.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
i = 0
outputstr=""
for item in self.pattern:
i += 1
if i < len(self.pattern):
if item == ".":
outputstr=outputstr+"dot-"
elif item == "_":
outputstr=outputstr+"dash-"
else:
if item == ".":
outputstr=outputstr+"dot"
elif item == "_":
outputstr=outputstr+"dash"
return outputstr
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
def __str__(self):
super().__str__()
1 Answer

Unsubscribed User
6,415 PointsHey man, your solution definitely does work. However, you're also adding extra code to the S
subclass (the definition of the __str__()
method). This code addition also throws an error if you try printing an instance of the S
subclass. Long story short, defining it is not necessary because since S
is a subclass of the Letter
class , it just uses Letter's __str__()
. As a side point, while your solution does work, you can achieve similar results using the following more succinct solution:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
str_list = []
for item in self.pattern:
if item == ".":
str_list.append("dot")
else:
str_list.append("dash")
return "-".join(str_list)
Péter Simon
1,054 PointsPéter Simon
1,054 PointsThank you, you're right about not needing the str() method in the subclass as it inherits.