Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial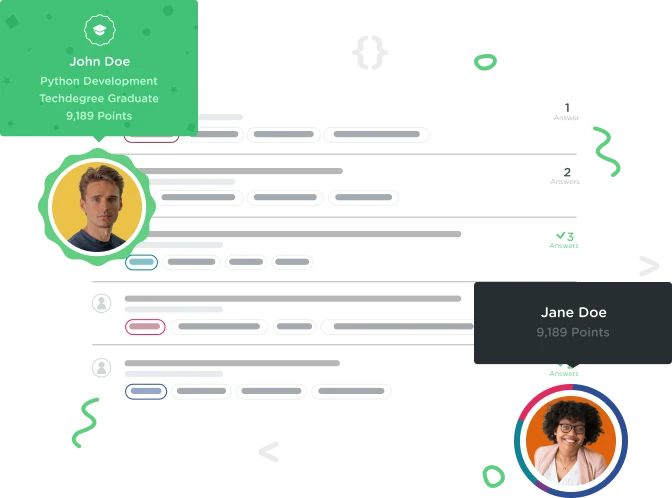
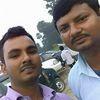
shubhamkt
11,675 Pointsmultidimensional array
how to print the multi dimensional array
<?php
//edit this array
$contacts = array(array ('name'=>'Alena Holligan', 'email'=>'alena.holligan@teamtreehouse.com'), array('name' =>'Dave McFarland','email' =>'dave.mcfarland@teamtreehouse.com')
, array('name'=>'Treasure Porth', 'email' =>'treasure.porth@teamtreehouse.com'), array('name'=>'Andrew Chalkley',
'email'=> 'andrew.chalkley@teamtreehouse.com'));
foreach ($contacts as $name)
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$name['name'][]</li>\n";
echo "</ul>\n";
3 Answers
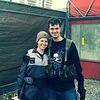
Christopher Parke
21,978 PointsI think it's because you have a syntax error in this line
"<li>$name['name'][]</li>\n";
should be "<li>$name['name']</li>\n";
because the [] is already there and includes 'name' as a parameter
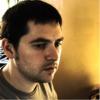
geoffrey
28,736 PointsTo print the content of the nested array, you need to loop through the arrays within the array. In other words, you need to use a foreach as you did.
If you don't find the solution, feel free to ask, but that's better you find it on your own.
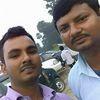
shubhamkt
11,675 Pointshelp with the solution.
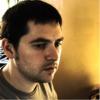
geoffrey
28,736 PointsShubham kumar Trivedi I've checked the code challenge.
I'm sorry because I gave you a wrong information, now I see clearly the issue. On your side, you wanted to use a foreach loop, (which is correct) to display the information you need.
Except that the foreach should have been done this way:
<?php
$contacts = array(
array("name"=>'Alena Holligan',"email"=>"alena.holligan@teamtreehouse.com"),
array("name"=>'Dave McFarland',"email"=>"dave.mcfarland@teamtreehouse.com"),
array("name"=>'Treasure Porth',"email"=>"treasure.porth@teamtreehouse.com"),
array("name"=>'Andrew Chalkley',"email"=>"andrew.chalkley@teamtreehouse.com")
);
echo "<ul>\n";
foreach($contacts as $contact)
{
echo"<li>".$contact["name"]." : ".$contact["email"]."</li>";
}
echo "<ul>\n";
If you click the preview tab within the code challenge, this will format correctly the result and will look good. However, this is not what they ask in the code challenge, they don't want us to create a foreach.
The error says that :
Bummer! ****** Example: $array[#][STRING]
What we have to do is simply access the elements of the nested array this way:
<?php
$contacts = array(
array("name"=>'Alena Holligan',"email"=>"alena.holligan@teamtreehouse.com"),
array("name"=>'Dave McFarland',"email"=>"dave.mcfarland@teamtreehouse.com"),
array("name"=>'Treasure Porth',"email"=>"treasure.porth@teamtreehouse.com"),
array("name"=>'Andrew Chalkley',"email"=>"andrew.chalkley@teamtreehouse.com")
);
echo "<ul>\n";
echo "<li>".$contacts[0]["name"]." : ".$contacts[0]["email"]."</li>\n"; // [0] => First array within contacts.
echo "<li>".$contacts[1]["name"]." : ".$contacts[1]["email"]."</li>\n"; // [1] => Second array within contacts and etc...
echo "<li>".$contacts[2]["name"]." : ".$contacts[2]["email"]."</li>\n";
echo "<li>".$contacts[3]["name"]." : ".$contacts[3]["email"]."</li>\n";
echo "</ul>\n";
However It's true that in a real situation you would use an array !
Hope It helps and sorry for the wrong information given at first, I read too quickly.