Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial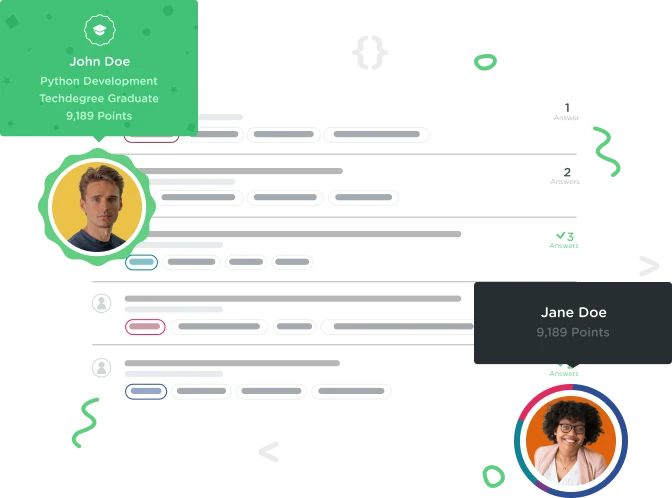

John Vicencio
6,413 PointsMultidimentional array (questioning the question)
Question: in the code below, we have a simple array of contact names. We want to use the $contacts array to fill in the hardcoded list of names an email addresses. To hold the email as well as the name for each contact, we need a multidimensional array, meaning each person in the contact list will have their own array. The first step is to make each person their own single item ASSOCIATIVE array, using 'name' as the key for these internal arrays.
My solution didn't work, I think because the question isn't really clear.
Don't I do this?
$contacts = array(
array('Alena Holligan', 'alena.holligan@teamtreehouse.com'),
array('Dave McFarland', 'dave.mcfarland@teamtreehouse.com'),
array('Treasure Porth', 'treasure.porth@teamtreehouse.com'),
array('Andrew Chalkley', 'andrew.chalkley@teamtreehouse.com')
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$contacts[0][0] : $contacts[0][1]</li>\n";
echo "<li>$contacts[1][0] : $contacts[1][1]</li>\n";
echo "<li>$contacts[2][0] : $contacts[2][1]</li>\n";
echo "<li>$contacts[3][0] : $contacts[3][2]</li>\n";
echo "</ul>\n";
But didn't work.
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
19 Answers
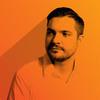
David Soards
18,368 Pointsthe challenges in this course have not been clear at all. it's very frustrating

Emilze Eloy
2,993 Points<?php
//edit this array
$contacts = array(
array(
"name"=>"Alena Holligan",
"email"=> 'alena.holligan@teamtreehouse.com'
),
array(
"name" => "Dave McFarland",
"email" => "dave.mcfarland@teamtreehouse.com"
),
array(
"name"=> "Treasure Porth",
"email"=>'treasure.porth@teamtreehouse.com'
),
array(
"name" => "Andrew Chalkley",
"email" => "andrew.chalkley@teamtreehouse.com"
)
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>". $contacts[0]['name'] . " : ". $contacts[0]['email'] ."</li>\n";
echo "<li>". $contacts[1]['name'] . " : ". $contacts[1]['email'] ."</li>\n";
echo "<li>". $contacts[2]['name'] . " : ". $contacts[2]['email'] ."</li>\n";
echo "<li>". $contacts[3]['name'] . " : ". $contacts[3]['email'] ."</li>\n";
echo "</ul>\n";
?>
Finally my code run correctly! :)
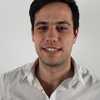
Juan Ignacio Lambardi
3,943 PointsThis is the third and last part of the challenge :)
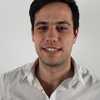
Juan Ignacio Lambardi
3,943 Points<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
$contacts = array (
array (
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
),
array (
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
),
array (
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
),
array (
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
),
);
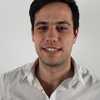
Juan Ignacio Lambardi
3,943 PointsThis is the second part of the challenge! :)
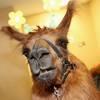
A X
12,842 PointsYes you answered with code, but you didn't clarify on what this question was asking...as I also agree with John that the question isn't worded clearly at all in terms of what's an acceptable answer and what isn't. How did you arrive at this as the "correct" answer (aka the one TH will accept)?
This also doesn't work for part 1 of the assignment as the OP is asking about.
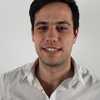
Juan Ignacio Lambardi
3,943 Points$contacts = array (
array (
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com',
),
array (
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com',
),
array (
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com',
),
array (
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com',
),
);
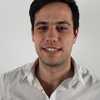
Juan Ignacio Lambardi
3,943 PointsHi Eveliina Champagne, your code it looks fine, maybe the editor of treehouse doesn't works fine if we use the "[]" to create a multidimensional array. Try using and replace the first part of the third part for the code i left above of this comment! :)
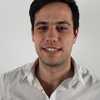
Juan Ignacio Lambardi
3,943 Points<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
$contacts = array (
array ( 'name' => 'Alena Holligan'),
array ( 'name' => 'Dave McFarland'),
array ( 'name' => 'Treasure Porth'),
array ( 'name' => 'Andrew Chalkley'),
);
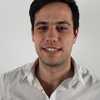
Juan Ignacio Lambardi
3,943 PointsThis is the first part of the challenge!

John Vicencio
6,413 PointsThanks Juan. I figured it out.
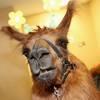
A X
12,842 PointsIt doesn't work for part 1 of the challenge. Your code results in an error message: "I do not see the required output. You should not be modifying the output at this point."
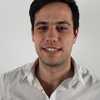
Juan Ignacio Lambardi
3,943 Points<?php
//edit this array
$contacts = array (
array (
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com',
),
array (
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com',
),
array (
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com',
),
array (
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com',
),
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>". $contacts [0] ['name'] . " : " . $contacts [0] ['email'] . "</li>\n";
echo "<li>". $contacts [1] ['name'] . " : " . $contacts [1] ['email'] . "</li>\n";
echo "<li>". $contacts [2] ['name'] . " : " . $contacts [2] ['email'] . "</li>\n";
echo "<li>". $contacts [3] ['name'] . " : " . $contacts [3] ['email'] . "</li>\n";
echo "</ul>\n";
?>

John Vicencio
6,413 PointsThanks I figured it out. I was doing 3 steps ahead but had to do one step at the time.

hassan alzahrani
3,623 PointsBTW the space after the array name need to be removed or the treehouse excersize will gave an error even it is working o0
This
$contacts [0] ['name']
to this
$contacts[0]['name']
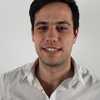
Juan Ignacio Lambardi
3,943 PointsHi Hassan, it's works to me as I showed. But maybe you're right. !
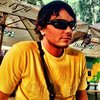
Tibor Ruzinyi
17,968 PointsAnother option(but not working as an answer):
<?php
//edit this array
$contacts["Alena"]=[
"name" => "Alena Holligans",
"email" => "alena.holligan@teamtreehouse.com"
];
$contacts["Dave"]=[
"name" => "Dave McFarland",
"email" => "dave.mcfarland@teamtreehouse.com"
];
$contacts["Treasure"]=[
"name" => "Treasure Porth",
"email" => "treasure.porth@teamtreehouse.com"
];
$contacts["Andrew"]=[
"name" => "Andrew Chalkley",
"email" => "andrew.chalkley@teamtreehouse.com"
];
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>" . $contacts["Alena"]["name"] . " : " . $contacts["Alena"]["email"] . "</li>\n";
echo "<li>" . $contacts["Dave"]["name"] . " : " . $contacts["Dave"]["email"] . "</li>\n";
echo "<li>" . $contacts["Treasure"]["name"] . " : " . $contacts["Treasure"]["email"] . "</li>\n";
echo "<li>" . $contacts["Andrew"]["name"] . " : " . $contacts["Andrew"]["email"] . "</li>\n";
echo "</ul>\n";
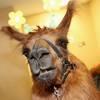
A X
12,842 PointsTibor, I thought double quotes are treated differently than single quotes in php...I'm not sure if that will effect the array or not, but it's more a question than anything else...
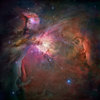
Matthew Pray
7,137 PointsIts sad that only treehouse version works.

John Vicencio
6,413 PointsThanks! I was a bit ahead haha.

Nasir Atta
2,177 Points<?php //edit this array $contacts[] = array ( 'name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com', ); $contacts[] =array ( 'name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com', ); $contacts[] =array ( 'name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com', ); $contacts[] = array ( 'name' => 'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com', ); //echo var_dump($contacts);
echo "<ul>\n"; echo "<li>".$contacts[0]['name']." : ".$contacts[0]['email']."</li>\n"; echo "<li>".$contacts[1]['name']." : ".$contacts[1]['email']."</li>\n"; echo "<li>".$contacts[2]['name']." : ".$contacts[2]['email']."</li>\n"; echo "<li>".$contacts[3]['name']." : ".$contacts[3]['email']."</li>\n"; echo "</ul>\n";
?>
make sure that " : " have space from both side between the double commas.

robbertdekock
13,390 PointsCan anyone tell me if this is the 'cleanest' way to write the first 2 parts of this exercise?
<?php
$contacts = [
['name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'],
['name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'],
['name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'],
['name' => 'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com'],
];
?>
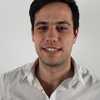
Juan Ignacio Lambardi
3,943 PointsHi Robert, your code it's ok!, it's clean. Although, you can use the "array()" and the result will be the same. Here is a very useful link http://www.php-fig.org/psr/

robbertdekock
13,390 Pointsthnx!

Eveliina Champagne
11,554 PointsHi Juan,
sorry about the messy post. I'm new to posting here. Thank you for helping me! :)

Mahmoud Helal
Full Stack JavaScript Techdegree Graduate 17,856 Points<?php $contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
$contacts = array ( array ( 'name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'), array ( 'name' => 'Dave McFarland','email' => 'dave.mcfarland@teamtreehouse.com'), array ( 'name' => 'Treasure Porth','email' => 'treasure.porth@teamtreehouse.com'), array ( 'name' => 'Andrew Chalkley','email' => 'andrew.chalkley@teamtreehouse.com') );
echo "<ul>\n"; echo "<li>" . $contacts[0]['name'], ' : ' . $contacts[0]['email'] . "</li>\n"; echo "<li>" . $contacts[1]['name'], ' : ' . $contacts[1]['email'] . "</li>\n"; echo "<li>" . $contacts[2]['name'], ' : ' . $contacts[2]['email'] . "</li>\n"; echo "<li>" . $contacts[3]['name'], ' : ' . $contacts[3]['email'] . "</li>\n"; echo "</ul>\n";

Tinashe Bvukumbwe
14,089 Points<?php //edit this array $contacts = array( array( "name"=>"Alena Holligan", "email"=> 'alena.holligan@teamtreehouse.com' ),
array( "name" => "Dave McFarland", "email" => "dave.mcfarland@teamtreehouse.com" ),
array(
"name"=> "Treasure Porth",
"email"=>'treasure.porth@teamtreehouse.com'
),
array(
"name" => "Andrew Chalkley",
"email" => "andrew.chalkley@teamtreehouse.com"
) );
echo "<ul>\n"; //$contacts[0] will return 'Alena Holligan' in our simple array of names. echo "<li>". $contacts[0]['name'] . " : ". $contacts[0]['email'] ."</li>\n"; echo "<li>". $contacts[1]['name'] . " : ". $contacts[1]['email'] ."</li>\n"; echo "<li>". $contacts[2]['name'] . " : ". $contacts[2]['email'] ."</li>\n"; echo "<li>". $contacts[3]['name'] . " : ". $contacts[3]['email'] ."</li>\n"; echo "</ul>\n";
?>
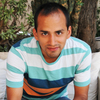
Cesar De La Vega
13,900 PointsThis worked for me:
<?php
//edit this array
$contacts = array(
[
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com',
],
[
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com',
],
[
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com',
],
[
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com',
],
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";

Christopher Hall
11,158 PointsHere is my solution
<?php
//edit this array
$contacts = [
["name" => "Alena Holligan",
"email" => "alena.holligan@teamtreehouse.com"],
["name" => "Dave McFarland",
"email" => "dave.mcfarland@teamtreehouse.com"],
["name" => "Treasure Porth",
"email" => "treasure.porth@teamtreehouse.com"],
["name" => "Andrew Chalkley",
"email" => "andrew.chalkley@teamtreehouse.com"]
];
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";

Eveliina Champagne
11,554 PointsHi,
I can't figure out what is wrong with this code. I'm on the third task of this challenge.
"Bummer! I do not see the use of the names from contacts array in the output. When calling the multidimensional array, you must first call the outer indexed array and then the inner associative array. Example: $array[#][STRING]"
<?php
//edit this array
$contacts [] = [
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com',
];
$contacts [] = [
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com',
];
$contacts [] = [
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com',
];
$contacts [] = [
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com',
];
echo "<ul>\n"; //$contacts[0] will return 'Alena Holligan' in our simple array of names. echo "<li>". $contacts [0]['name'] . " : " . $contacts [0] ['email'] . "</li>\n"; echo "<li>". $contacts [1] ['name'] . " : " . $contacts [1] ['email'] . "</li>\n"; echo "<li>". $contacts [2] ['name'] . " : " . $contacts [2] ['email'] . "</li>\n"; echo "<li>". $contacts [3] ['name'] . " : " . $contacts [3] ['email'] . "</li>\n"; echo "</ul>\n";
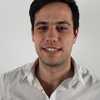
Juan Ignacio Lambardi
3,943 PointsHi Eveliina, to see it better insert your code after writting... ```php so it'll appear with the black background! :)
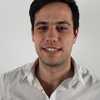
Juan Ignacio Lambardi
3,943 PointsMy pleasure!, and sorry for my english jaja

Eric C
7,998 PointsJuan can you elaborate on how to post code with the black background, I don't understand. Thanks in advance!
Joseph Arquímedes Collado Tineo
1,942 PointsJoseph Arquímedes Collado Tineo
1,942 PointsThis is another option: