Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial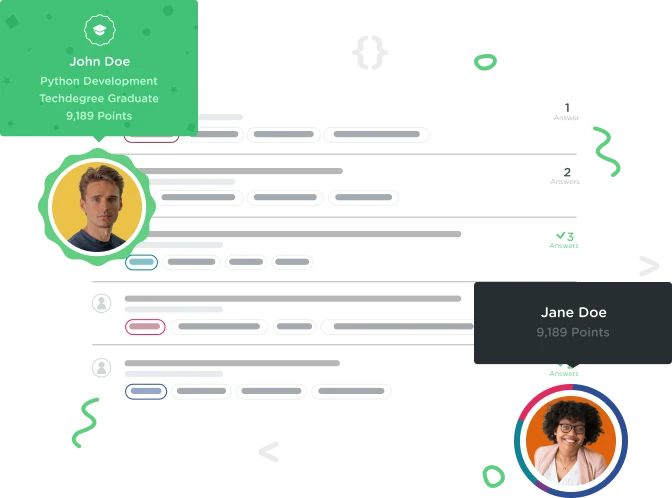

C. Kattenberg
3,652 PointsMySQL queries with PHP
I'm learning how to use databases in combination with PHP, and I came across this example that shows how to connect to a database with the mysqli_connect() function, and then issue a query with the mysqli_query() function:
<?php
//Step1
$db = mysqli_connect('localhost','root','root','database_name')
or die('Error connecting to MySQL server.');
?>
<html>
<head>
</head>
<body>
<h1>PHP connect to MySQL</h1>
<?php
//Step2
$query = "SELECT * FROM table_name";
mysqli_query($db, $query) or die('Error querying database.');
//Step3
$result = mysqli_query($db, $query);
$row = mysqli_fetch_array($result);
while ($row = mysqli_fetch_array($result)) {
echo $row['first_name'] . ' ' . $row['last_name'] . ': ' . $row['email'] . ' ' . $row['city'] .'<br />';
}
//Step 4
mysqli_close($db);
?>
</body>
</html>
I don't really understand why the connection variable ($db) has to be passed to the mysqli_query() function. The connection was already made in the beginning, right? Is this in case there is a connection with multiple databases?
2 Answers
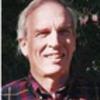
jcorum
71,830 PointsIn step 1 you create a connection and give it a name: $db.
In step 2 you create a SQL query statement, and then pass it and the connection to mysqli_query.
Think of it this way. You could create connections to several different databases: $db1, $db2, etc.
Then, when running queries, you could run one query against $db1 and another against $db2.
So creating the connection and using it are separate steps.
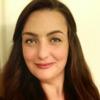
Jennifer Nordell
Treehouse TeacherAs I understand it it's because it's saving that connection in that variable. If you didn't, you'd have to retype that entire line after the $db declaration every time you use it. So everywhere you see $db you'd have had to type out that whooooooole thing. That would be annoying.
The connection takes the parameters of the host, user name, password, and the name of the database. Also you could set up another connection to another database that you could store in $db2 for example. And the mysqli_query takes the parameters of the connection and the SQL string and (optionally) a resultMode. The connection is passed in because it's required by the function. Here's the documentation. http://php.net/manual/en/mysqli.query.php
C. Kattenberg
3,652 PointsC. Kattenberg
3,652 PointsOk. Thanks. One follow up question:
Would the code also work if, instead of creating the $db variable at the beginning, you were to type out the connect function into the query function like this:
or is this invalid, because the connection is not made, initially?